Advanced Contract Programming Example: SchellingCoin
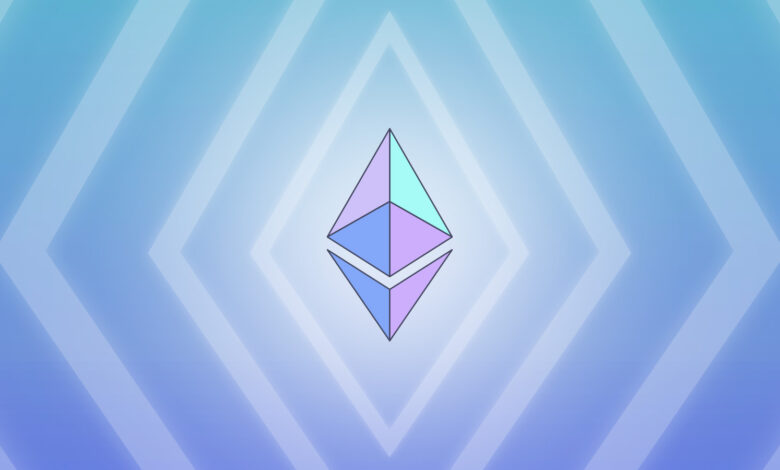
Writing efficient decentralized functions in Ethereum is on the identical time straightforward and exhausting. The simple half everyone knows: reasonably than needing to create your personal blockchain, handle difficult database code, cope with networking and NAT traversal, or any of the opposite complexities involving writing a peer-to-peer app from scratch, you possibly can write code in a easy, high-level programming language like Serpent or Mutan (or LLL for those who favor mucking round a bit lower-level), with the simplicity of a toy scripting language however the energy and safety of a full blockchain backing it up. A complete implementation of a fundamental identify registry will be carried out in two strains of code that embody the important logic of this system: if not contract.storage[msg.data[0]]: contract.storage[msg.data[0]] = msg.knowledge[1]. Use the zeroth knowledge merchandise within the message as a key and the primary as a worth; if the hot button is not but taken then set the important thing to the specified worth. A telephone e book you can add entries to, however the place entries, as soon as made, can’t be modified. Nevertheless, there may be additionally a tough half: decentralized functions are more likely to contain logic that’s essentially advanced, and there’s no means that any simplifications to the programming surroundings can ever take away that reality (nonetheless, libraries constructed on prime of the programming language may alleviate particular points). Moreover, any dapps doing something actually fascinating is more likely to contain cryptographic protocols and economics, and everyone knows how advanced these are.
The aim of this text might be to undergo a contract that is a vital element of a totally decentralized cryptoeconomic ecosystem: a decentralized oracle. The oracle might be applied utilizing the SchellingCoin protocol, described in a earlier weblog publish. The core concept behind the protocol is that everybody “votes” on a specific worth (on this case, we’ll use wei per US cent for instance, as that may find yourself very helpful in monetary contracts), and everybody who submitted a vote that’s between the twenty fifth and 75 percentile (ie. near median) receives a reward. The median is taken to be the “true value”. So as to improve safety, every spherical is finished through a two-step dedication protocol: within the first part, everybody selects a worth P which is the worth they are going to be voting for, and submits H = sha3([msg.sender, P]) to the contract, and within the second part everybody submits the P that they chose and the contract accepts solely these values that match the beforehand supplied hash. Rewarding and analysis is then carried out on the finish.
The rationale why it really works is that this. In the course of the first part, everyone seems to be so to talk “in the dark”; they have no idea what the others might be submitting, seeing maybe solely hashes of different votes. The one data they’ve is that they’re purported to be submitting the value of a US cent in wei. Thus, figuring out solely that the one worth that different individuals’s solutions are going to be biased in the direction of is the precise wei/UScent, the rational option to vote for to be able to maximize one’s likelihood of being near-median is the wei/UScent itself. Therefore, it is in everybody’s finest pursuits to come back collectively and all present their finest estimate of the wei/UScent value. An fascinating philosophical level is that that is additionally the identical means that proof-of-work blockchains work, besides that in that case what you might be voting on is the time order of transactions as a substitute of some specific numeric worth; this reasonably strongly means that this protocol is more likely to be viable a minimum of for some functions.
In fact, in actuality numerous sorts of particular eventualities and assaults are attainable, and the truth that the value of any asset is very often managed by a small variety of centralized exchanges makes issues tougher. For instance, one possible failure mode is that if there’s a market share cut up between the BTC/USD on Bitstamp, Bitfinex and MtGox, and MtGox is the most well-liked alternate, then the incentives may drive all of the votes to combination across the GOX-BTC/USD value particularly, and at that time it’s solely unclear what would occur when MtGox will get hacked and the value on that alternate alone, and never the others, falls to $100. Everybody could nicely find yourself following their particular person incentives and sticking to one another to the protocol’s collective doom. The way to cope with these conditions and whether or not or not they’re even important is a completely empirical challenge; it’s exhausting to say what the actual world will do beforehand.
Formalizing the protocol, we have now the next:
- Each set of N blocks (right here, we set N = 100) constitutes a separate “epoch”. We outline the epoch quantity as ground(block.quantity / 100), and we outline the block quantity modulo 100 to be the “residual”.
- If the residual is lower than 50, then anybody can submit a transaction with any worth V and hash H = sha3([msg.sender, R, P]), the place P is their estimate of the value of 1 US cent in wei (keep in mind, 1 wei = 10-18 ether, and 1 cent = 10-2 USD) and R is a random quantity.
- If the residual is bigger than 50, then anybody who submitted a hash can submit P, and the contract will test if sha3([msg.sender, P]) matches the hash.
- On the finish of the epoch (or, extra exactly, on the level of the primary “ping” through the subsequent epoch), everybody who submitted a worth for P between the twenty fifth and seventy fifth percentile, weighted by deposit, will get their deposit again plus a small reward, everybody else will get their deposit minus a small penalty, and the median worth is taken to be the true UScent/wei value. Everybody who did not submit a legitimate worth for P will get their deposit again minus a small penalty.
Word that there are attainable optimizations to the protocol; for instance, one may introduce a characteristic that permits anybody with a specific
P
worth to steal the deposit from whoever submitted the hash, making it impractical to share one’s
P
to attempt to affect individuals’s votes earlier than residual 50 hits and the second part begins. Nevertheless, to maintain this instance from getting too difficult we won’t do that; moreover, I personally am skeptical of “forced private data revelation” methods normally as a result of I predict that a lot of them will change into ineffective with the eventual introduction of generalized zero-knowledge proofs, fully homomorphic encryption and obfuscation. For instance, one may think an attacker beating such a scheme by supplying a zero-knowledge proof that their
P
worth is inside a specific 1015 wei-wide vary, giving sufficient data to offer customers a goal however not sufficient to virtually find the precise worth of
P
. Given these issues, and given the need for simplicity, for now the straightforward two-round protocol with no bells-and-whistles is finest.
Earlier than we begin coding SchellingCoin itself, there may be one different contract that we might want to create: a sorting operate. The one strategy to calculate the median of an inventory of numbers and decide who’s in a specific percentile vary is to type the listing, so we’ll need a generalized operate to do this. For added utility, we’ll make our sorting operate generic: we’ll type pairs as a substitute of integers. Thus, for examples, [30, 1, 90, 2, 70, 3, 50, 4] would change into [ 30, 1, 50, 4, 70, 3, 90, 2 ]. Utilizing this operate, one can type an inventory containing any form of object just by making an array of pairs the place the primary quantity is the important thing to type by and the second quantity is a pointer to the article in mum or dad reminiscence or storage. This is the code:
if msg.datasize == 0: return([], 0) else: low = array(msg.datasize) lsz = 0 excessive = array(msg.datasize) hsz = 0 i = 2 whereas i < msg.datasize: if msg.knowledge[i] < msg.knowledge[0]: low[lsz] = msg.knowledge[i] low[lsz + 1] = msg.knowledge[i + 1] lsz += 2 else: excessive[hsz] = msg.knowledge[i] excessive[hsz + 1] = msg.knowledge[i + 1] hsz += 2 i = i + 2 low = name(contract.handle, low, lsz, lsz) excessive = name(contract.handle, excessive, hsz, hsz) o = array(msg.datasize) i = 0 whereas i < lsz: o[i] = low[i] i += 1 o[lsz] = msg.knowledge[0] o[lsz + 1] = msg.knowledge[1] j = 0 whereas j < hsz: o[lsz + 2 + j] = excessive[j] j += 1 return(o, msg.datasize)
Pc college students could acknowledge this as a quicksort implementation; the concept is that we first cut up the listing into two, with one half containing all the pieces lower than the primary merchandise and the opposite half containing all the pieces better, then we recursively type the primary and second lists (the recursion terminates ultimately, since ultimately the sub-lists could have zero or one objects, during which case we simply return these values instantly), and at last we concatenate output = sorted_less_than_list + first merchandise + sorted_greater_than_list and return that array. Now, placing that into “quicksort_pairs.se”, let’s construct the code for the actual SchellingCoin. Be happy to go to the github to see the code multi function piece; right here, we’ll undergo it just a few strains at a time.
First, some initialization code:
init: contract.storage[0] = block.quantity contract.storage[3] = create('quicksort_pairs.se') code: HASHES = 2^160 VALUES = 2^170
The primary code block units contract storage index 0 to the present block quantity at initialization time, after which creates a quicksort contract and saves that in storage index 3. Word that theoretically you’d wish to simply create the quicksort contract as soon as and consult with it by handle; we’re simply doing an inline create for simplicity and to indicate the characteristic. Within the code we begin off by declaring two variables to function pseudo-constants; HASHES = 2160 because the pointer for the place we retailer hashes, and VALUES = 2170 because the pointer for the place we retailer values from the second part.
Now, from right here let’s skip to the underside half of the code, as a result of that seems to be extra handy and it is the code that really will get run “first” over the course of the contract’s lifetime.
# Hash submission if msg.knowledge[0] == 1: if block.quantity % 100 < 50: cur = contract.storage[1] pos = HASHES + cur * 3 contract.storage[pos] = msg.knowledge[1] contract.storage[pos + 1] = msg.worth contract.storage[pos + 2] = msg.sender contract.storage[1] = cur + 1 return(cur) # Worth submission elif msg.knowledge[0] == 2: if sha3([msg.sender, msg.data[3], msg.knowledge[2]], 2) == contract.storage[HASHES + msg.data[1] * 3]: contract.storage[VALUES + msg.data[1]] = msg.knowledge[2] return(1) # Steadiness request elif msg.knowledge[0] == 3: return(contract.stability) # Worth request else: return(contract.storage[2])
The primary essential paradigm that we see right here is utilizing msg.knowledge[0] to consult with a “message type”; messages with zeroth knowledge merchandise 1 are hash submissions, 2 are worth submissions, 3 are stability requests and 4 are requests for the present UScent/wei value. This can be a customary interface that you’ll probably see throughout very many contracts. The primary clause, the one for submitting hashes, is considerably concerned, so allow us to break it down step-by-step. The first function right here is to permit individuals to submit hashes, and file submissions in storage. To that finish, the contract is storing the information sequentially in storage beginning at index 2160. We have to retailer three items of knowledge – the precise hash, the scale of the accompanying deposit, and the sender handle, for every hash, so we try this. We additionally use storage index 1 to retailer what number of hashes have already been submitted. Thus, if two hashes have been submitted, storage will look one thing like this:
The exact directions within the clause are:
- Proceed provided that the residual is lower than 50.
- Set the variable cur to storage index 1, the place we’re going to be storing the variety of hashes which have already been submitted
- Set the variable pos to the index in storage during which we might be placing the brand new hash
- Save the hash (provided as the primary knowledge merchandise), the sender handle and the worth in storage
- Set the brand new variety of hashes to cur + 1
- Return the index of the hash provided
Technically, if the one customers of SchellingCoin are individuals, step 5 is pointless; though the index might be vital in a later step, a wise consumer might doubtlessly merely scan the
cur
variable instantly after the transaction, eradicating the necessity for the opcodes wanted to deal with the return. Nevertheless, since we anticipate that in Ethereum we could have loads of situations of contracts utilizing different contracts, we’ll present the return worth as a behavior of excellent machine interface.
The subsequent clause is for submitting values. Right here, we ask for 2 knowledge objects as enter: the index the place the hash was saved throughout step one of the protocol (that is the return worth of the earlier clause), and the precise worth. We then hash the sender and worth collectively, and if the hash matches then we save the lead to one other place in contract storage; another method is to make use of one single beginning storage location and easily have 4 slots per hash as a substitute of three. We return 1 is profitable, and nothing for a failure. The third and fourth clauses are merely trivial knowledge requests; the third is a stability test, and the fourth returns the contract’s present view of the value.
That is all for the interface aspect of the contract; nonetheless, the one half that we nonetheless have to do is the half that really aggregates the votes. We’ll break that up into components. First, we have now:
HASHES = 2^160 VALUES = 2^170 if block.quantity / 100 > contract.storage[0] / 100: # Type all hashes N = contract.storage[1] o = array(N) i = 0 j = 0 whereas i < N: if contract.storage[VALUES + i]: o[j] = contract.storage[VALUES + i] o[j + 1] = i j += 2 i += 1 values = name(contract.storage[3], o, j, j)
First, we use storage index 0 to retailer the final accessed epoch, and we test if the present epoch is greater than the final accessed epoch. Whether it is, then that indicators the beginning of a brand new epoch, so we have to course of all of the votes and clear the contract for the subsequent epoch. We begin off by copying the values which have been submitted to an array (values that haven’t been submitted, ie. zeroes, usually are not put into this array). We preserve two operating counters, i and j; the counter i runs by way of all worth slots, however the counter j counts solely the worth slots which have one thing inside them. Word that the array that we produce is of the shape [ val1, index1, val2, index2 … ], the place index1 and so on are the indices of the related values within the authentic values array in contract storage, thus for instance, the next values would result in the next array:
Then, we ship that array by way of the quicksort contract, which types knowledge pairs within the array. After the kind, we find yourself with:
Now, what we have now is a sorted listing of all of the values that individuals have submitted, alongside tips to the place the related metadata is saved in chilly storage. The subsequent a part of the code will deal with three issues concurrently. First, it can compute the entire quantity that has been deposited; that is helpful in determining the median. Second, we’ll make two arrays to signify deposits and their related addresses, and we’ll take away that knowledge from the contract. Lastly, we’ll 99.9% refund anybody who didn’t submit a worth. Theoretically, we might make it a 70% refund or a 0% refund, however which may make the contract too dangerous for individuals to throw their life financial savings in (which is definitely what we wish in a proof-of-stake-weighted system; the extra ether is thrown in by respectable customers the tougher it’s for an attacker to muster sufficient funds to launch an assault). here is the code; be happy to grasp every line your self:
# Calculate whole deposit, refund non-submitters and # cleanup deposits = array(j / 2) addresses = array(j / 2) i = 0 total_deposit = 0 whereas i < j / 2: base_index = HASHES + values[i * 2 + 1] * 3 contract.storage[base_index] = 0 deposits[i] = contract.storage[base_index + 1] contract.storage[base_index + 1] = 0 addresses[i] = contract.storage[base_index + 2] contract.storage[base_index + 2] = 0 if contract.storage[VALUES + values[i * 2 + 1]]: total_deposit += deposits[i] else: ship(addresses[i], deposits[i] * 999 / 1000) i += 1
Now, we come to the final a part of the code, the half the computes the median and rewards individuals. In line with the specification, we have to reward everybody between the twenty fifth and seventy fifth percentile, and take the median (ie. fiftieth percentile) as the reality. To truly do that, we wanted to first type the information; now that the information is sorted, nonetheless, it is so simple as sustaining a operating counter of “total deposited value of everything in the list up to this point”. If that worth is between 25% and 75% of the entire deposit, then we ship a reward barely better than what they despatched in, in any other case we ship a barely smaller reward. Right here is the code:
inverse_profit_ratio = total_deposit / (contract.stability / 1000) + 1 # Reward everybody i = 0 running_deposit_sum = 0 halfway_passed = 0 whereas i < j / 2: new_deposit_sum = running_deposit_sum + deposits[i] if new_deposit_sum > total_deposit / 4 and running_deposit_sum < total_deposit * 3 / 4: ship(addresses[i], deposits[i] + deposits[i] / inverse_profit_ratio * 3) else: ship(addresses[i], deposits[i] - deposits[i] / inverse_profit_ratio) if not halfway_passed and new_deposit_sum > total_deposit / 2: contract.storage[2] = contract.storage[VALUES + i] halfway_passed = 1 contract.storage[VALUES + i] = 0 running_deposit_sum = new_deposit_sum i += 1 contract.storage[0] = block.quantity contract.storage[1] = 0
On the identical time, you possibly can see we additionally zero out the values in contract storage, and we replace the epoch and reset the variety of hashes to zero. The primary worth that we calculate, the “inverse profit ratio”, is principally the inverse of the “interest rate” you get in your deposit; if inverse_profit_ratio = 33333, and also you submitted 1000000 wei, then you definitely get 1000090 wei again in case you are near the median and 999970 in case you are not (ie. your anticipated return is 1000030 wei). Word that though this quantity is tiny, it occurs per hundred blocks, so actually it’s fairly massive. And that is all there may be to it. If you wish to take a look at, then attempt operating the next Python script:
import pyethereum t = pyethereum.tester s = t.state() s.mine(123) c = s.contract('schellingcoin.se') c2 = s.contract('schellinghelper.se') vals = [[125, 200], [126, 900], [127, 500], [128, 300], [133, 300], [135, 150], [135, 150]] s.ship(t.k9, c, 10**15) print "Submitting hashes" for i, v in enumerate(vals): print s.ship(t.keys[i], c, v[1], [1] + s.ship(t.keys[i], c2, 0, [v[0], 12378971241241])) s.mine(50) print "Submitting vals" for i, v in enumerate(vals): if i != 5: print s.ship(t.keys[i], c, 0, [2, i, v[0], 12378971241241]) else: print s.ship(t.keys[i], c, 0, [2, i, 4]) print "Final check" s.mine(50) print s.ship(t.k9, c, 0, [4])
Earlier than operating the script, be sure you fill the ‘schellinghelper.se’ file with return(sha3([msg.sender, msg.data[0], msg.knowledge[1]], 3)); right here, we’re simply being lazy and utilizing Serpent itself to assist us put the hash collectively; in actuality, this could positively be carried out off-chain. In case you try this, and run the script, the final worth printed by the contract ought to return 127.
Word that this contract because it stands isn’t actually scalable by itself; at 1000+ customers, whoever provides the primary transaction in the beginning of every epoch would want to pay a really great amount of gasoline. The best way to repair this economically is in fact to reward the submitter of the transaction, and take a flat charge off each participant to pay for the reward. Additionally, nonetheless, the rate of interest per epoch is tiny, so it might already not be value it for customers to take part until they’ve a signigicant amount of money, and the flat charge could make this drawback even worse.
To permit individuals to take part with small quantities of ether, the only answer is to create a “stake pool” the place individuals put their ether right into a contract for the long run, after which the pool votes collectively, randomly choosing a participant weighted by stake to provide the worth to vote for in every epoch. This would scale back the load from two transactions per consumer per epoch to a few transactions per pool per epoch (eg. 1 pool = 1000 customers) plus one transaction per consumer to deposit/withdraw. Word that, not like Bitcoin mining swimming pools, this stake pool is totally decentralized and blockchain-based, so it introduces at most very small centralization dangers. Nevertheless, that is an instructive instance to indicate how a single contract or DAO could find yourself resulting in a complete ecosystem of infrastructure engaged on the blockchain with contracts speaking to one another; a specialised SchellingCoin blockchain wouldn’t be capable of invent pooling mechanisms after the actual fact and combine them so effectively.
So far as functions go, essentially the most speedy one is contracts for distinction, and ultimately a decentralized cryptographic US greenback; if you wish to see an try at such a contract see here, though that code is nearly actually susceptible to market manipulation assaults (purchase a really great amount of USD contained in the system, then purchase USD available on the market to maneuver the value 0.5%, then promote the USD contained in the system for a fast 0.3% revenue). The core concept behind the decentralized crypto-dollar is easy: have a financial institution with two currencies, USD and ether (or reasonably, UScent and wei), with the power to have a optimistic or adverse amount of {dollars}, and manipulate the rate of interest on greenback deposits to be able to preserve the contract’s internet greenback publicity at all times near zero in order that the contract doesn’t have any internet obligations in currencies that it doesn’t have the power to carry. An easier method would merely be to have an expanding-supply foreign money that adjusts its provide operate to focus on the USD, however that’s problematic as a result of there isn’t a safety if the worth falls an excessive amount of. These sorts of functions, nonetheless, will probably take fairly a very long time (in crypto phrases; fairly quick in conventional finance phrases in fact) to get constructed.