Upgradable Smart Contracts
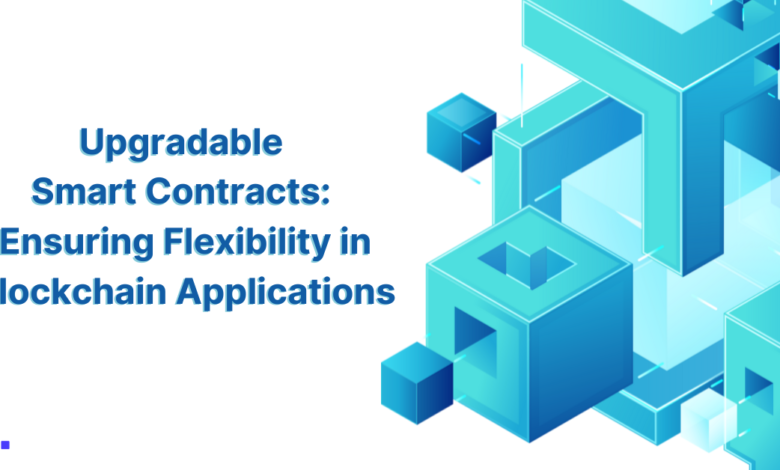
What are Upgradable Smart Contracts?
Upgrading good contracts refers back to the skill to change or prolong the performance of a deployed good contract with out disrupting the present system or requiring customers to work together with a brand new contract. That is significantly difficult as a result of immutable nature of blockchain, the place deployed contracts can’t be modified. Upgradable good contracts clear up this by permitting modifications whereas sustaining the identical contract tackle, thus preserving state and consumer interactions.
Why Do We Want Upgradable Smart Contracts?
- Bug Fixes and Safety Patches: Smart contracts, like all software program, can have bugs or vulnerabilities found post-deployment. Upgrades permit these points to be addressed with out requiring customers to change to a brand new contract.
- Function Enhancements: As dApps evolve, new options or enhancements could also be wanted. Upgradable contracts allow including these enhancements seamlessly.
- Compliance and Rules: Regulatory environments can change, necessitating updates to contract logic to make sure ongoing compliance.
- Consumer Expertise: Customers can proceed interacting with the identical contract tackle, avoiding the confusion and potential lack of funds related to contract migrations.
Kinds of Upgradable Smart Contracts
1. Not Actually Upgrading (Parametrizing Every thing)
This strategy includes designing contracts with parameters that may be adjusted with out altering the contract code.
Professionals:
- Easy to implement.
- No complicated improve mechanisms are required.
Cons:
- Restricted flexibility as future modifications have to be anticipated on the time of preliminary deployment.
- Can result in complicated and inefficient contract design as a result of extreme parametrization.
2. Social Migration
Social migration includes deploying a brand new contract model and inspiring customers emigrate their interactions to the brand new contract voluntarily.
Professionals:
- Totally decentralized and clear as customers select emigrate.
- No central authority is required to handle the improve.
Cons:
- Threat of consumer fragmentation, the place some customers don’t migrate, resulting in divided consumer bases.
- Coordination challenges and potential lack of consumer funds through the migration course of.
3. Proxies
Proxies contain a proxy contract that delegates calls to an implementation contract, permitting the implementation to be swapped out as wanted.
Professionals:
- Versatile and highly effective, enabling complete upgrades with out redeploying the contract.
- Customers proceed interacting with the unique contract tackle.
Cons:
- Advanced to implement and keep.
- Safety dangers similar to storage clashes and performance selector conflicts.
Issues with Proxies
Storage Clashes
Storage clashes happen when the storage format of the proxy contract conflicts with that of the implementation contract. Every slot in a contract’s storage is assigned a singular index, and if each the proxy and the implementation contracts use the identical storage slots for various variables, it can lead to corrupted knowledge.
Instance:
contract Proxy {
tackle implementation;
uint256 proxyData; // Proxy-specific knowledge
operate upgradeTo(tackle _implementation) exterior {
implementation = _implementation;
}
fallback() exterior payable {
(bool success, ) = implementation.delegatecall(msg.knowledge);
require(success);
}
}
contract ImplementationV1 {
uint256 knowledge;
operate setData(uint256 _data) exterior {
knowledge = _data;
}
operate getData() exterior view returns (uint256) {
return knowledge;
}
}
If ImplementationV1 is changed with one other implementation that makes use of the identical storage slots in another way, knowledge may be overwritten, resulting in storage clashes.
Perform Selector Conflicts
Perform selector conflicts happen when totally different capabilities within the proxy and implementation contracts have the identical signature, which is the primary 4 bytes of the Keccak-256 hash of the operate’s prototype. In Solidity, every operate is recognized by a singular selector, but when two capabilities in several contracts have the identical selector, it could possibly result in conflicts when delegatecall is used.
Let’s delve into an in depth instance to know this concern.
Take into account the next proxy contract and two implementation contracts:
Proxy Contract:
contract Proxy {
tackle public implementation;
operate upgradeTo(tackle _implementation) exterior {
implementation = _implementation;
}
fallback() exterior payable {
(bool success, ) = implementation.delegatecall(msg.knowledge);
require(success);
}
}
Implementation Contract V1:
contract ImplementationV1 {
uint256 public knowledge;
operate setData(uint256 _data) exterior {
knowledge = _data;
}
}
Implementation Contract V2:
contract ImplementationV2 {
uint256 public knowledge;
operate setData(uint256 _data) exterior {
knowledge = _data;
}
operate additionalFunction() exterior view returns (string reminiscence) {
return "This is V2";
}
}
On this situation, each ImplementationV1 and ImplementationV2 have a operate named setData, which generates the identical operate selector. If the proxy is initially utilizing ImplementationV1 after which upgraded to ImplementationV2, calls to setData will accurately delegate to the brand new implementation.
Nonetheless, if the proxy itself had a operate with the identical selector as setData, it might trigger a battle.
Proxy Contract with Conflicting Perform:
contract Proxy {
tackle public implementation;
operate upgradeTo(tackle _implementation) exterior {
implementation = _implementation;
}
operate setData(uint256 _data) exterior {
// This operate would battle with Implementation contracts' setData
}
fallback() exterior payable {
(bool success, ) = implementation.delegatecall(msg.knowledge);
require(success);
}
}
What Occurs Throughout a Battle?
When setData is known as on the proxy contract, Solidity will test the operate selectors to find out which operate to execute. Because the proxy contract itself has a operate setData with the identical selector, it can execute the proxy’s setData operate as a substitute of delegating the decision to the implementation contract.
Because of this the meant name to ImplementationV1 or ImplementationV2’s setData operate won’t ever happen, resulting in surprising conduct and potential bugs.
Technical Rationalization:
- Perform Selector Era: The operate selector is generated as the primary 4 bytes of the Keccak-256 hash of the operate prototype. For instance, setData(uint256) generates a singular selector.
- Proxy Fallback Mechanism: When a name is made to the proxy, the fallback operate makes use of delegatecall to ahead the decision to the implementation contract.
- Battle Decision: If the proxy contract has a operate with the identical selector, Solidity’s operate dispatch mechanism will prioritize the operate within the proxy contract over the implementation contract.
To keep away from such conflicts, it’s essential to make sure that the proxy contract doesn’t have any capabilities that might battle with these within the implementation contracts. Correct naming conventions and cautious contract design might help mitigate these points.
What’s DELEGATECALL?
DELEGATECALL is a low-level operate in Solidity that permits a contract to execute code from one other contract whereas preserving the unique context (e.g., msg.sender and msg.worth). That is important for proxy patterns, the place the proxy contract delegates operate calls to the implementation contract.
Delegate Name vs Name Perform
Much like a name operate, delegatecall is a elementary characteristic of Ethereum. Nonetheless, they work a bit in another way. Consider delegatecall as a name choice that permits one contract to borrow a operate from one other contract.
As an instance this, let’s have a look at an instance utilizing Solidity – an object-oriented programming language for writing good contracts.
contract B {
// NOTE: storage format have to be the identical as contract A
uint256 public num;
tackle public sender;
uint256 public worth;
operate setVars(uint256 _num) public payable {
num = _num;
sender = msg.sender;
worth = msg.worth;
}
}
Contract B has three storage variables (num, sender, and worth), and one operate setVars that updates our num worth. In Ethereum, contract storage variables are saved in a selected storage knowledge construction that’s listed ranging from zero. Because of this num is at index zero, sender at index one, and worth at index two.
Now, let’s deploy one other contract – Contract A. This one additionally has a setVars operate. Nonetheless, it makes a delegatecall to Contract B.
contract A {
uint256 public num;
tackle public sender;
uint256 public worth;
operate setVars(tackle _contract, uint256 _num) public payable {
// A's storage is about, B is just not modified.
// (bool success, bytes reminiscence knowledge) = _contract.delegatecall(
(bool success, ) = _contract.delegatecall(
abi.encodeWithSignature("setVars(uint256)", _num)
);
if (!success) {
revert("delegatecall failed");
}
}
}
Usually, if Contract A known as setVars on Contract B, it might solely replace Contract B’s num storage. Nonetheless, through the use of delegatecall, it says “call setVars function and then pass _num as an input parameter but call it in our contract (A).” In essence, it ‘borrows’ the setVars operate and makes use of it in its personal context.
Understanding Storage in DELEGATECALL
It’s fascinating to see how delegatecall works with storage on a deeper stage. The borrowed operate (setVars of Contract B) doesn’t have a look at the names of the storage variables of the calling contract (Contract A) however as a substitute, at their storage slots.
If we used the setVars operate from Contract B utilizing delegatecall, the primary storage slot (which is num in Contract A) will probably be up to date as a substitute of num in Contract B, and so forth.
One different vital facet to recollect is that the info sort of the storage slots in Contract A doesn’t need to match that of Contract B. Even when they’re totally different, delegatecall works by simply updating the storage slot of the contract making the decision.
On this means, delegatecall allows Contract A to successfully make the most of the logic of Contract B whereas working inside its personal storage context.
What’s EIP1967?
EIP1967 is an Ethereum Enchancment Proposal that standardizes the storage slots utilized by proxy contracts to keep away from storage clashes. It defines particular storage slots for implementation addresses, making certain compatibility and stability throughout totally different implementations.
Instance of OpenZeppelin Minimalistic Proxy
To construct a minimalistic proxy utilizing EIP1967, let’s comply with these steps:
Step 1 – Constructing the Implementation Contract
We’ll begin by making a dummy contract ImplementationA. This contract can have a uint256 public worth and a operate to set the worth.
contract ImplementationA {
uint256 public worth;
operate setValue(uint256 newValue) public {
worth = newValue;
}
}
Step 2 – Making a Helper Perform
To simply encode the operate name knowledge, we’ll create a helper operate named getDataToTransact.
operate getDataToTransact(uint256 numberToUpdate) public pure returns (bytes reminiscence) {
return abi.encodeWithSignature("setValue(uint256)", numberToUpdate);
}
Step 3 – Studying the Proxy
Subsequent, we create a operate in Solidity named readStorage to learn our storage within the proxy.
operate readStorage() public view returns (uint256 valueAtStorageSlotZero) {
meeting {
valueAtStorageSlotZero := sload(0)
}
}
Step 4 – Deployment and Upgrading
Deploy our proxy and ImplementationA. Let’s seize ImplementationA’s tackle and set it within the proxy.
Step 5 – The Core Logic
Once we name the proxy with knowledge, it delegates the decision to ImplementationA and saves the storage within the proxy tackle.
contract EIP1967Proxy {
bytes32 non-public fixed _IMPLEMENTATION_SLOT = keccak256("eip1967.proxy.implementation");
constructor(tackle _logic) {
bytes32 slot = _IMPLEMENTATION_SLOT;
meeting {
sstore(slot, _logic)
}
}
fallback() exterior payable {
meeting {
let impl := sload(_IMPLEMENTATION_SLOT)
calldatacopy(0, 0, calldatasize())
let consequence := delegatecall(gasoline(), impl, 0, calldatasize(), 0, 0)
returndatacopy(0, 0, returndatasize())
change consequence
case 0 { revert(0, returndatasize()) }
default { return(0, returndatasize()) }
}
}
operate setImplementation(tackle newImplementation) public {
bytes32 slot = _IMPLEMENTATION_SLOT;
meeting {
sstore(slot, newImplementation)
}
}
}
Step 6 – Isometrics
To make sure that our logic works accurately, we’ll learn the output from the readStorage operate. We’ll then create a brand new implementation contract ImplementationB.
contract ImplementationB {
uint256 public worth;
operate setValue(uint256 newValue) public {
worth = newValue + 2;
}
}
After deploying ImplementationB and updating the proxy, calling the proxy ought to now delegate calls to ImplementationB, reflecting the brand new logic.
Kinds of Proxies and Their Professionals and Cons
Clear Proxy
This contract implements a proxy that’s upgradeable by an admin.
To keep away from proxy selector clashing, which may probably be utilized in an assault, this contract makes use of the clear proxy sample. This sample implies two issues that go hand in hand:
- If any account apart from the admin calls the proxy, the decision will probably be forwarded to the implementation, even when that decision matches one of many admin capabilities uncovered by the proxy itself.
- If the admin calls the proxy, it could possibly entry the admin capabilities, however its calls won’t ever be forwarded to the implementation. If the admin tries to name a operate on the implementation, it can fail with an error that claims “admin cannot fallback to proxy target”.
These properties imply that the admin account can solely be used for admin actions like upgrading the proxy or altering the admin, so it’s greatest if it’s a devoted account that’s not used for anything. This can keep away from complications as a result of sudden errors when attempting to name a operate from the proxy implementation.
UUPS (Common Upgradeable Proxy Normal)
UUPS works equally to the Clear Proxy Sample. We use msg.sender as a key in the identical means as within the beforehand defined sample. The one distinction is the place we put the operate to improve the logic’s contract: within the proxy or within the logic. Within the Clear Proxy Sample, the operate to improve is within the proxy’s contract, and the way in which to alter the logic seems to be the identical for all logic contracts.
It’s modified in UUPS. The operate to improve to a brand new model is applied within the logic’s contract, so the mechanism of upgrading may change over time. Furthermore, if the brand new model of the logic doesn’t have the upgrading mechanism, the entire undertaking will probably be immutable and received’t be capable of change. Due to this fact, if you want to make use of this sample, you ought to be very cautious to not by chance take from your self the choice to improve out.
Learn extra on Transparent vs UUPS Proxies.
Instance of UUPS Proxy Implementation Utilizing EIP1967Proxy
BoxV1.sol:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
import {OwnableUpgradeable} from "@openzeppelin/contracts-upgradeable/access/OwnableUpgradeable.sol";
import {Initializable} from "@openzeppelin/contracts-upgradeable/proxy/utils/Initializable.sol";
import {UUPSUpgradeable} from "@openzeppelin/contracts-upgradeable/proxy/utils/UUPSUpgradeable.sol";
contract BoxV1 is Initializable, OwnableUpgradeable, UUPSUpgradeable {
uint256 inner worth;
/// @customized:oz-upgrades-unsafe-allow constructor
constructor() {
_disableInitializers();
}
operate initialize() public initializer {
__Ownable_init();
__UUPSUpgradeable_init();
}
operate getValue() public view returns (uint256) {
return worth;
}
operate model() public pure returns (uint256) {
return 1;
}
operate _authorizeUpgrade(tackle newImplementation) inner override onlyOwner {}
}
BoxV2.sol:
/// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
import {OwnableUpgradeable} from "@openzeppelin/contracts-upgradeable/access/OwnableUpgradeable.sol";
import {Initializable} from "@openzeppelin/contracts-upgradeable/proxy/utils/Initializable.sol";
import {UUPSUpgradeable} from "@openzeppelin/contracts-upgradeable/proxy/utils/UUPSUpgradeable.sol";
contract BoxV2 is Initializable, OwnableUpgradeable, UUPSUpgradeable {
uint256 inner worth;
/// @customized:oz-upgrades-unsafe-allow constructor
constructor() {
_disableInitializers();
}
operate initialize() public initializer {
__Ownable_init();
__UUPSUpgradeable_init();
}
operate setValue(uint256 newValue) public {
worth = newValue;
}
operate getValue() public view returns (uint256) {
return worth;
}
operate model() public pure returns (uint256) {
return 2;
}
operate _authorizeUpgrade(
tackle newImplementation
) inner override onlyOwner {}
}
EIP1967Proxy.sol:
// SPDX-License-Identifier: MIT
// OpenZeppelin Contracts (final up to date v5.0.0) (proxy/ERC1967/ERC1967Proxy.sol)
pragma solidity ^0.8.20;
import {Proxy} from "../Proxy.sol";
import {ERC1967Utils} from "./ERC1967Utils.sol";
/**
* @dev This contract implements an upgradeable proxy. It's upgradeable as a result of calls are delegated to an
* implementation tackle that may be modified. This tackle is saved in storage within the location specified by
* https://eips.ethereum.org/EIPS/eip-1967[ERC-1967], in order that it would not battle with the storage format of the
* implementation behind the proxy.
*/
contract ERC1967Proxy is Proxy {
constructor(tackle implementation, bytes reminiscence _data) payable {
ERC1967Utils.upgradeToAndCall(implementation, _data);
}
operate _implementation() inner view digital override returns (tackle) {
return ERC1967Utils.getImplementation();
}
}
Deploy and Improve Course of:
- Deploy BoxV1.
- Deploy EIP1967Proxy with the tackle of BoxV1.
- Work together with BoxV1 via the proxy.
- Deploy BoxV2.
- Improve the proxy to make use of BoxV2.
BoxV1 field = new BoxV1();
ERC1967Proxy proxy = new ERC1967Proxy(tackle(field), "");
BoxV2 newBox = new BoxV2();
BoxV1 proxy = BoxV1(payable(proxyAddress));
proxy.upgradeTo(tackle(newBox));
Why Ought to We Keep away from Upgradable Smart Contracts?
- Complexity: The added complexity in growth, testing, and auditing can introduce new vulnerabilities.
- Gasoline Prices: Proxy mechanisms can improve gasoline prices, impacting the effectivity of the contract.
- Safety Dangers: Improperly managed upgrades can result in safety breaches and lack of funds.
- Centralization: Improve mechanisms typically introduce a central level of management, which may be at odds with the decentralized ethos of blockchain.
Upgradable good contracts supply a strong software for sustaining and bettering blockchain purposes. Nonetheless, they arrive with their very own set of challenges and trade-offs. Builders should rigorously contemplate the need of upgradability, weigh the professionals and cons of various approaches, and implement strong testing and safety measures to make sure the integrity of their methods. Whereas upgradability gives flexibility, it have to be balanced with the foundational rules of safety and decentralization.
Web3 Labs has experience in good contract growth. Be at liberty to achieve out for any help concerning good contract growth, gasoline optimizations, auditing or safety of good contracts, or any consultations.