Create an On-Chain Wallet Tracker with Web3 Alerts
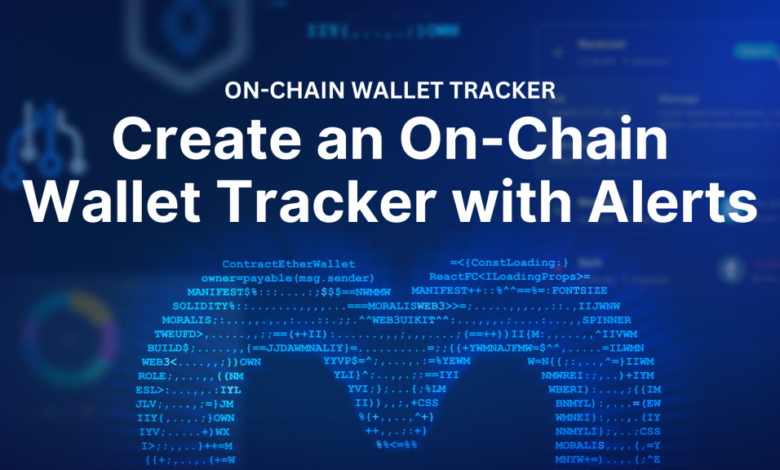
An on-chain pockets tracker is a instrument that lets you monitor the exercise of any crypto tackle in real-time. Nonetheless, from a standard perspective, constantly monitoring wallets and blockchain networks for related occasions has been fairly cumbersome. Thankfully, because of the Moralis Streams API, that is not the case!
With the Streams API, you possibly can effortlessly arrange streams to observe any tackle on any community, permitting you to create your personal on-chain pockets tracker in a heartbeat. To spotlight the accessibility of this instrument, right here’s an instance of learn how to arrange a stream monitoring a pockets’s native on-chain transactions on the Sepolia testnet:
async operate streams(){ const choices = { chains: [EvmChain.SEPOLIA], tag: "transfers", description: "Listen to Transfers", includeContractLogs: false, includeNativeTxs: true, webhookUrl: "replace_me" } const newStream = await Moralis.Streams.add(choices) const {id} = newStream.toJSON(); const tackle = "0xa50981073aB67555c14F4640CEeB5D3efC5c7af2"; await Moralis.Streams.addAddress({tackle, id}) } streams()
For a extra detailed breakdown of how this works and the way you should utilize the Streams API to construct an on-chain pockets tracker, be part of us on this tutorial or try the Moralis YouTube video under!
Additionally, to make use of the Streams API, you need to have a Moralis account. Thankfully, you possibly can join with Moralis completely without cost, and also you’ll get quick entry to all our industry-leading improvement instruments!
Overview
In as we speak’s article, we’ll begin by explaining the ins and outs of on-chain pockets trackers. From there, we’ll soar straight into our on-chain pockets tracker tutorial and present you learn how to monitor a pockets in 5 easy steps utilizing Moralis’ Streams API:
- Create a Moralis Account
- Spin Up a Server
- Set Up a Moralis Stream
- Run the Script
- Obtain Alerts
Lastly, to high issues off, we’ll dive deeper into Moralis and discover different instruments past the Streams API, together with one of the best API for crypto costs, our free NFT API, and plenty of others! However, with out additional delay, let’s begin by answering the query, “What is an on-chain wallet tracker?”.
What’s an On-Chain Wallet Tracker?
On-chain pockets trackers are instruments that assist customers observe the exercise of explicit wallets. What’s extra, one of the best pockets trackers often present alert options, instantly notifying customers of essential on-chain occasions as quickly as they occur so that they don’t miss a beat!
There are numerous sorts of on-chain pockets trackers, reminiscent of a token portfolio tracker, and most of them have their very own set of distinctive options. Furthermore, some solely present fundamental data, such because the transaction quantity, whereas others give customers extra detailed information, together with all concerned addresses, block data, logs, and way more.
All in all, the core performance of an on-chain pockets tracker is to offer a clear and real-time view of pockets exercise!
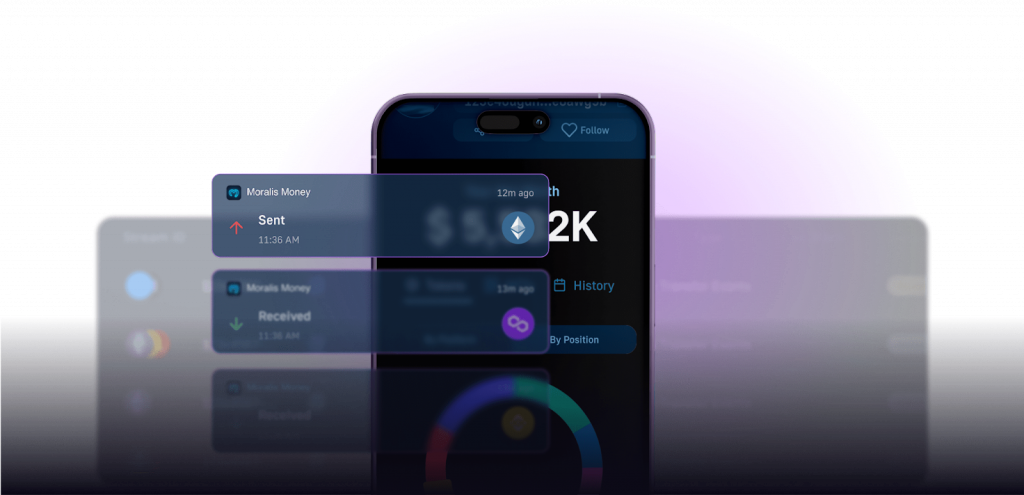
So, how does this work?
An on-chain pockets tracker constantly displays related addresses and blockchain networks. As quickly as they detect related on-chain exercise, they notify customers, giving them updates in real-time. Nonetheless, organising this technique is less complicated stated than carried out, so distinguished pockets trackers leverage Web3 APIs to simplify the workflow!
However what’s the finest API for constructing an on-chain pockets tracker? And the way does it work? For the solutions to those questions, be part of us within the subsequent part as we present you the simplest technique to monitor any pockets tackle utilizing Moralis’ Streams API!
On-Chain Wallet Tracker Tutorial: How one can Monitor Any Tackle in 5 Steps
The simplest technique to monitor any crypto tackle and construct an on-chain pockets tracker is to make use of Moralis’ Streams API. With the Streams API, you possibly can seamlessly arrange alerts to get notified about Web3 occasions mechanically. Moreover, this interface helps over 44 million addresses and contracts throughout all main blockchain networks, together with Ethereum, Polygon, BNB Sensible Chain (BSC), and plenty of others!
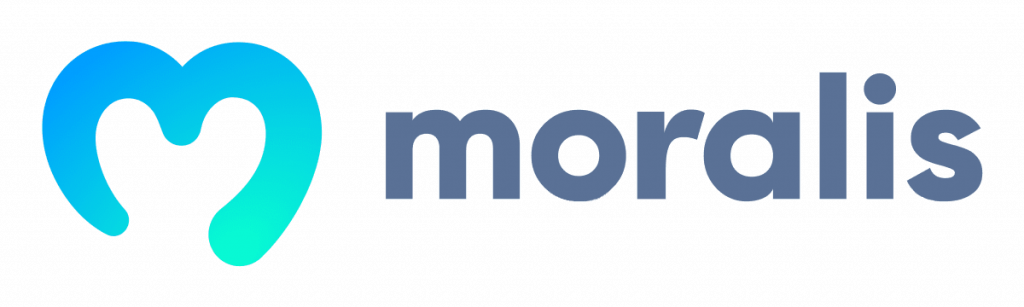
So, how does the Streams API work?
With the Streams API, you possibly can seamlessly arrange your personal personalized streams to get real-time, on-chain occasions despatched instantly to your challenge’s backend by way of Web3 webhooks!
However to raised clarify how this works, we’ll merely present you learn how to monitor a pockets tackle in 5 easy steps:
- Create a Moralis Account
- Spin Up a Server
- Set Up a Moralis Stream
- Run the Script
- Obtain Alerts
Now, earlier than we will soar into the preliminary step of this tutorial, you want to deal with a couple of stipulations!
Stipulations
Whereas the Moralis SDK and Streams API assist a number of programming languages, this tutorial will present you learn how to arrange a Moralis stream utilizing JavaScript. We’ll additionally use ngrok to get a webhook URL. Consequently, earlier than you proceed, be sure to have the next prepared:
- Node.js v.14+
- NPM/Yarn
- Ngrok
Step 1: Create a Moralis Account
To make use of the Streams API, you need to have a Moralis API key. And to get an API key, you want to join with Moralis. As such, in the event you haven’t already, click on on the ”Begin for Free” button on the high proper to arrange an account:

With an account at hand, head on over to the ”Settings” tab, scroll all the way down to the ”API Keys” part, and replica your API key:
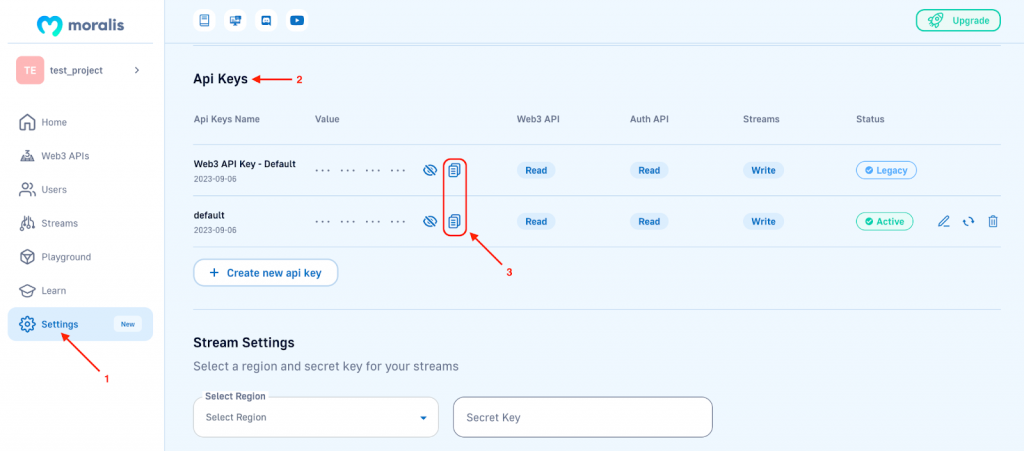
Preserve the important thing for now, as you’ll want it within the third step of this tutorial!
Step 2: Spin Up a Server
For the second step, you want to arrange a server that can obtain the Moralis webhooks from the Streams API. And for this, we’ll be utilizing a easy Node.js Categorical app!
So, to kickstart the second step of this tutorial, begin by organising a brand new challenge in your built-in improvement atmosphere (IDE) and putting in Categorical. From there, create a brand new ”index.js” file within the challenge’s root folder and add the next code:
const categorical = require("express"); const app = categorical(); const port = 3000; app.use(categorical.json()); app.put up("/webhook", async (req, res) => { const {physique} = req; strive { console.log(physique); } catch (e) { console.log(e); return res.standing(400).json(); } return res.standing(200).json(); }); app.hear(port, () => { console.log(`Listening to streams`); });
Within the code, we outline a single endpoint referred to as /webhook
, to which Moralis can put up the streams. Right here, we parse the physique that Moralis sends and log the response within the console:
app.put up("/webhook", async (req, res) => { const {physique} = req; strive { console.log(physique); } catch (e) { console.log(e); return res.standing(400).json(); } return res.standing(200).json(); });
Subsequent, now you can spin up the server by working the next command within the challenge’s root folder:
node index.js
In response, you must now see ”Listening to streams” logged in your console:

From right here, open a brand new terminal and run the command under to make use of ngrok to open a brand new tunnel to ”port 3000”, the place our server is presently working:
ngrok http http://localhost:3000
Executing this command will present you one thing that appears like this, the place you possibly can go forward and replica your webhook URL:
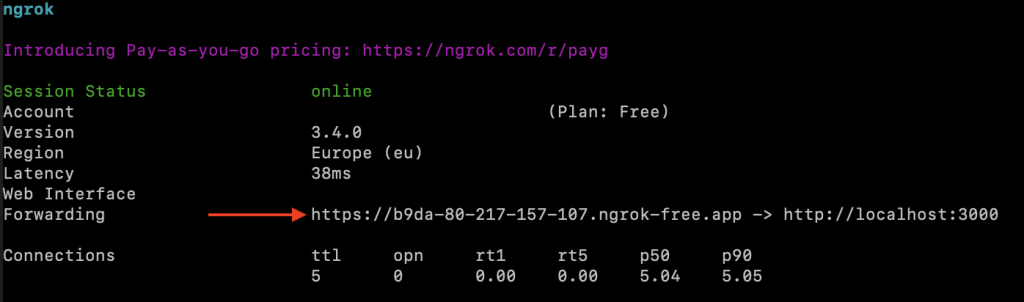
Save the URL for now, as you’ll want it within the subsequent step!
Step 3: Set Up a Moralis Stream
With a server up and working, you’re now able to create a Moralis stream. To take action, open a brand new window in your IDE and create a challenge folder. From there, go forward and arrange a brand new ”.env” file and add your Moralis API key as an atmosphere variable. It ought to look one thing like this:
MORALIS_KEY=’YOUR_API_KEY’
Subsequent, create a brand new ”index.js” file and add the next code:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/common-evm-utils"); require("dotenv").config(); Moralis.begin({ apiKey: course of.env.MORALIS_KEY, }); async operate streams(){ const choices = { chains: [EvmChain.SEPOLIA], tag: "transfers", description: "Listen to Transfers", includeContractLogs: false, includeNativeTxs: true, webhookUrl: "replace_me" } const newStream = await Moralis.Streams.add(choices) const {id} = newStream.toJSON(); const tackle = "0xa50981073aB67555c14F4640CEeB5D3efC5c7af2"; await Moralis.Streams.addAddress({tackle, id}) console.log("Stream successfully created") } streams()
Within the preliminary a part of the code, we begin by importing Moralis and our surroundings variable:
const Moralis = require("moralis").default; const { EvmChain } = require("@moralisweb3/common-evm-utils"); require("dotenv").config();
From there, we initialize the Moralis SDK utilizing our API key:
Moralis.begin({ apiKey: course of.env.MORALIS_KEY, });
We then arrange a streams()
operate the place we create an choices
object. Right here, we specify a couple of parameters, together with chains
, description
, tag
, what occasions to hear for, and our webhook URL:
async operate streams(){ const choices = { chains: [EvmChain.SEPOLIA], tag: "transfers", description: "Listen to Transfers", includeContractLogs: false, includeNativeTxs: true, webhookUrl: "replace_me" } //… }
Bear in mind to exchange replace_me
above with the webhook URL you copied within the earlier step. And don’t overlook to append it with /webhook
on the finish.
Subsequent, we create a brand new Moralis stream by calling the Streams.add()
technique whereas passing our choices
object as a parameter:
const newStream = await Moralis.Streams.add(choices)
From right here, we then fetch the id
of the stream and create an tackle
const to which we add the tackle we wish to take heed to:
const {id} = newStream.toJSON(); const tackle = "0xa50981073aB67555c14F4640CEeB5D3efC5c7af2";
Lastly, we add the tackle to our newly created stream utilizing the tackle
and id
constants:
await Moralis.Streams.addAddress({tackle, id})
And that’s it; we at the moment are able to run the script to create the stream!
Be aware: the Streams API helps all main EVM chains. So, if you wish to monitor addresses on different networks moreover Sepolia, you merely have to configure the chain
parameter of the choices
object above.
Step 4: Run the Script
Earlier than working the script, you want to set up a few dependencies. As such, run the next command in your challenge’s root folder:
npm set up moralis @moralisweb3/common-evm-utils dotenv
As soon as they’ve been put in, run this command to execute the code:
node index.js
If all the things labored as meant, you must obtain a message stating ”Stream efficiently created” in your console:

You also needs to get an empty webhook despatched to your server:
{ abi: [], block: { quantity: '', hash: '', timestamp: '' }, txs: [], txsInternal: [], logs: [], chainId: '', confirmed: true, retries: 0, tag: '', streamId: '', erc20Approvals: [], erc20Transfers: [], nftTokenApprovals: [], nftApprovals: { ERC721: [], ERC1155: [] }, nftTransfers: [], nativeBalances: [] }
And that’s it; you may have now efficiently arrange your personal stream utilizing Moralis’ Streams API!
Within the subsequent part, we’ll present you the way it works and what the response would possibly seem like!
Step 5: Obtain Alerts
Together with your stream up and working, you’ll obtain webhooks at any time when on-chain occasions happen based mostly in your specs. As such, for the stream created on this tutorial, you’ll get a real-time response at any time when the required pockets sends a local transaction on the Sepolia testnet, and it’ll look one thing like this:
{ confirmed: true, chainId: '0xaa36a7', abi: [], streamId: 'a6c08210-c7dc-416c-a140-4746478530ce', tag: 'transfers', retries: 0, block: { quantity: '4692430', hash: '0x31754432aa984e524472abb52c05f3175471ae2cb209a751f31e75a9dfab6a94', timestamp: '1699967412' }, logs: [], txs: [ { hash: '0x2fc3678670ee9895dc5dfc5f189f9839ab5c7351905b6d2b419518a5334450a2', gas: '21000', gasPrice: '1639464455', nonce: '9', input: '0x', transactionIndex: '78', fromAddress: '0xa50981073ab67555c14f4640ceeb5d3efc5c7af2', toAddress: '0xb76f252c8477818799e244ff68dee3b1e6b0ace5', value: '100000000000000', type: '2', //… receiptCumulativeGasUsed: '25084154', receiptGasUsed: '21000', receiptContractAddress: null, receiptRoot: null, receiptStatus: '1' } ], //… }
This response comprises a transaction hash, to and from addresses, the worth of the transaction, and way more. With this data, you possibly can seamlessly create an on-chain pockets tracker that alerts your customers in real-time at any time when one thing essential occurs!
To study extra about this wonderful instrument and learn how to customise your streams additional, try the official Streams API documentation web page!
Past the Streams API and Constructing an On-Chain Wallet Tracker – Exploring Moralis Additional
Moralis is an industry-leading Web3 API supplier, and our suite of premier improvement instruments lets you construct decentralized functions (dapps) each sooner and smarter. Consequently, when leveraging Moralis, it can save you numerous improvement time and assets!

Our complete suite of Web3 APIs consists of many interfaces you possibly can mix with the Streams API to construct refined initiatives. And down under, you’ll find three distinguished examples:
- NFT API: The NFT API is the {industry}’s premier instrument for NFT information. With this interface, you possibly can seamlessly fetch NFT balances, transactions, pricing information, and way more with solely single traces of code.
- Token API: With the Token API, you possibly can effortlessly get and combine token costs, pockets balances, transfers, and way more into your dapps. Consequently, when working with the Token API, it has by no means been simpler to construct all the things from decentralized exchanges to Web3 wallets.
- Wallet API: The Wallet API is one other useful gizmo you possibly can mix with the Streams API to construct a robust on-chain pockets tracker. This instrument helps over 500 million addresses throughout all main EVM chains, permitting you to seamlessly get native balances, NFTs, transfers, profile information, and extra from any tackle.
Additionally, all Moralis APIs are totally cross-chain appropriate. Consequently, when working with our instruments, you possibly can construct Web3 initiatives on a number of blockchains, together with Ethereum, BNB Sensible Chain, Polygon, Chiliz, Gnosis, and plenty of different networks!
If you wish to discover all our interfaces, try the Web3 API web page!
Abstract: How one can Create an On-Chain Wallet Tracker
In as we speak’s article, we kicked issues off by exploring the ins and outs of on-chain pockets trackers. In doing so, we realized that they’re crypto monitoring tools permitting customers to observe the exercise of a crypto tackle. Nonetheless, we additionally realized that monitoring blockchain networks and addresses is tough with out correct instruments, which is why we launched you to Moralis’ Streams API!
From there, we dove straight into our crypto pockets monitoring tutorial and confirmed you learn how to monitor a pockets in 5 steps utilizing the Streams API:
- Create a Moralis Account
- Spin Up a Server
- Set Up a Moralis Stream
- Run the Script
- Obtain Alerts
Consequently, when you’ve got adopted alongside this far, you now know learn how to monitor any tackle on any blockchain community with Moralis. Together with your newly acquired expertise, you possibly can seamlessly construct a real-time, on-chain pockets tracker software in a heartbeat!
By combining the Streams API with our different industry-leading interfaces, you possibly can seamlessly construct extra refined initiatives. Some distinguished examples embody the NFT API, Token API, Wallet API, and plenty of others.
Should you appreciated this tutorial on learn how to construct an on-chain pockets tracker, contemplate testing extra Web3 content material. As an example, study all you want to learn about meta transactions or try our Base faucet information! Additionally, don’t overlook to hitch Moralis as we speak. You’ll be able to create your account completely without cost, and also you’ll acquire quick entry to our industry-leading improvement instruments!