Gas Optimization Techniques for Solidity Smart Contracts
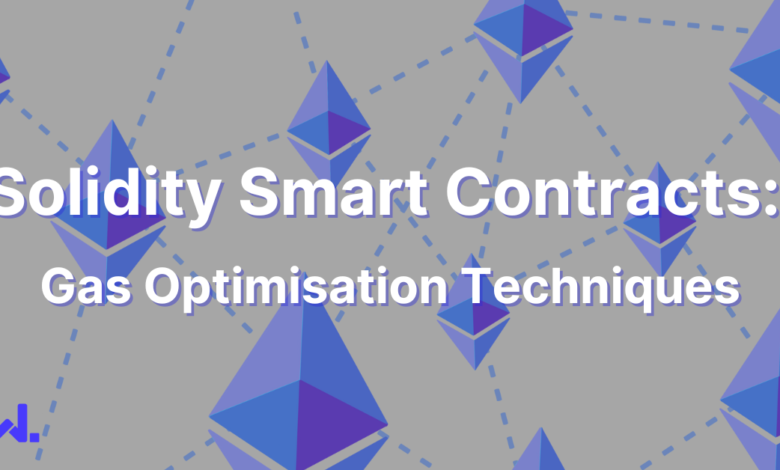
Final month, I printed an article highlighting how builders can considerably cut back fuel prices by choosing the proper storage varieties of their Solidity sensible contracts. This subject garnered appreciable curiosity, underscoring the continued developer quest for extra gas-efficient contract operations. As the recognition of Ethereum Digital Machine (EVM) networks continues to rise, so does the significance of minimizing transaction charges to make Web3 purposes extra accessible and cost-effective.
On this follow-up article, I’ll proceed exploring fuel optimization strategies in Solidity sensible contracts. Past storage sort choice, there are quite a few different methods builders can make use of to boost the effectivity of their sensible contracts. By implementing these strategies, builders cannot solely decrease fuel charges but in addition enhance the general efficiency and consumer expertise of their decentralized purposes (DApps). The pursuit of fuel optimization is essential for the scalability and sustainability of EVM networks, making it a key space of focus for the way forward for Web3 growth.
Gas Optimization Techniques
1. Storage areas
As mentioned within the earlier article, deciding on the suitable storage sort is an important place to begin for optimizing fuel prices in blockchain operations. The Ethereum Digital Machine (EVM) gives 5 storage areas: storage, reminiscence, calldata, stack, and logs. For extra particulars, please try my earlier article on Optimizing Gas in Solidity Smart Contracts. The approaches mentioned there spotlight some great benefits of utilizing reminiscence over storage. In a sensible instance, avoiding extreme studying and writing to storage can cut back fuel prices by as much as half!
2. Constants and Immutable variables
Let’s take the next sensible contact for instance:
contract GasComparison {
uint256 public worth = 250;
handle public account;
constructor() {
account = msg.sender;
}
}
The price for creating this contract is 174,049 fuel. As we are able to see, we’re utilizing storage with the occasion variables. To keep away from this, we must always refactor to make use of constants and immutable variables.
Constants and immutables are added on to the bytecode of the sensible contract after compilation, so they don’t use storage.
The optimized model of the earlier sensible contract is:
contract GasComparison {
uint256 public fixed VALUE = 250;
handle public immutable i_account;
constructor() {
i_account = msg.sender;
}
}
This time, the price of creating the sensible contract is 129154 fuel, 25% lower than the preliminary worth.
3. Personal over public variables
Persevering with with the earlier instance, we discover that occasion variables are public, which is problematic for two causes. First, it violates information encapsulation. Second, it generates extra bytecode for the getter operate, growing the general contract measurement. A bigger contract measurement means larger deployment prices as a result of the fuel price for deployment is proportional to the dimensions of the contract.
One solution to optimize is:
contract GasComparison {
uint256 non-public fixed VALUE = 250;
handle non-public immutable i_account;
constructor() {
i_account = msg.sender;
}
operate getValue() public pure returns (uint256) {
return VALUE;
}
}
Making all variables non-public with out offering getter features would make the sensible contract much less useful, as the info would not be accessible.
Even on this case, the creation price was diminished to 92,289 fuel, 28% decrease than the earlier case and 46% decrease than the primary case!
P.S. If we had saved the VALUE variable public and didn’t add the getValue operate, the identical quantity of fuel would have been consumed at contract creation.
4. Use interfaces
Utilizing interfaces in Solidity can considerably cut back the general measurement of your sensible contract’s compiled bytecode, as interfaces don’t embody the implementation of their features. This leads to a smaller contract measurement, which in flip lowers deployment prices since fuel prices for deployment are proportional to the contract measurement.
Moreover, calling features via interfaces will be extra gas-efficient. Since interfaces solely embody operate signatures, the bytecode for these calls will be optimized. This optimization results in potential fuel financial savings in comparison with calling features outlined instantly inside a bigger contract that accommodates extra logic and state.
Whereas utilizing interfaces will be helpful for advanced sensible contracts and features, it might not all the time be advantageous for less complicated contracts. Within the instance mentioned in earlier sections, including an interface can really improve fuel prices for easy contracts.
5. Inheritance over composition
Persevering with the interface concept we get to inheritance. Have a look at the next sensible contracts:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.18;
contract Worker {
handle public account;
constructor() {
account = msg.sender;
}
}
contract Supervisor {
Worker non-public worker;
constructor(handle _employeeAddress) {
worker = Worker(_employeeAddress);
}
operate getEmployeeAccount() exterior view returns (handle) {
return worker.account();
}
}
contract Executable {
Supervisor public supervisor;
constructor(handle _employeeAddress) {
supervisor = new Supervisor(_employeeAddress);
}
operate getMangerAccount() exterior view returns (handle) {
return supervisor.getEmployeeAccount();
}
}
Right here we now have 2 sensible contracts which work together via composition. The use-case is much less vital; what I wish to underline is the exterior name which Supervisor must make to get the Worker account. The getManagerAccount referred to as from the Executable account will eat 13,545 fuel.
We will optimise this through the use of inheritance:
contract Worker {
handle public account;
constructor() {
account = msg.sender;
}
}
contract Supervisor is Worker{
}
contract Executable {
Supervisor public supervisor;
constructor(){
supervisor = new Supervisor();
}
operate getMangerAccount() exterior view returns (handle) {
return supervisor.account();
}
}
This time getManagerAccount will take solely 8,014 fuel, 40% lower than the earlier case!
6. Variables measurement
Bytes and integers are among the many mostly used variable varieties in Solidity. Though the Ethereum Digital Machine (EVM) operates with 32-byte lengths, deciding on variables of this size for each occasion will not be excellent if the objective is fuel optimization.
Bytes
Let’s check out the next sensible contract:
contract BytesComparison {
bytes32 public fixed LONG_MESSAGE="Hello, world! This is a longer .";
bytes32 public fixed MEDIUM_MESSAGE="Hello, world!";
bytes32 public fixed SHORT_MESSAGE="H";
operate concatenateBytes32() public pure returns (bytes reminiscence) {
bytes reminiscence concatenated = new bytes(32 * 3);
for (uint i = 0; i < 32; i++) {
concatenated[i] = LONG_MESSAGE[i];
}
for (uint j = 0; j < 32; j++) {
concatenated[32 + j] = MEDIUM_MESSAGE[j];
}
for (uint ok = 0; ok < 32; ok++) {
concatenated[64 + k] = SHORT_MESSAGE[k];
}
return concatenated;
}
}
The execution price of the concatenateBytes32 is 28,909 fuel.
When it comes to fuel, optimization is really helpful when working with bytes to slim the dimensions to the worth used. On this case, an optimised model of this contract could be:
contract BytesComparison {
bytes32 public fixed LONG_MESSAGE="Hello, world! This is a longer .";
bytes16 public fixed MEDIUM_MESSAGE="Hello, world!";
bytes1 public fixed SHORT_MESSAGE="H";
operate concatenateBytes() public pure returns (bytes reminiscence) {
// Create a bytes array to carry the concatenated outcome
bytes reminiscence concatenated = new bytes(32 + 16 + 1);
for (uint i = 0; i < 32; i++) {
concatenated[i] = LONG_MESSAGE[i];
}
for (uint j = 0; j < 16; j++) {
concatenated[32 + j] = MEDIUM_MESSAGE[j];
}
concatenated[32 + 16] = SHORT_MESSAGE[0];
return concatenated;
}
}
On this case, the execution of concatenateBytes is 12,011 fuel, 59% decrease than within the earlier case.
Int
Nevertheless, this doesn’t apply to integer varieties. Whereas it may appear that utilizing int16 could be extra gas-efficient than int256, this isn’t the case. When coping with integer variables, it is suggested to make use of the 256-bit variations: int256 and uint256.
The Ethereum Digital Machine (EVM) works with 256-bit phrase measurement. Declaring them in several sizes would require Solidity to do extra operations to include them in 256-bit phrase measurement, leading to extra fuel consumption.
Let’s check out the next easy sensible contract:
contract IntComparison {
int128 public a=-55;
uint256 public b=2;
uint8 public c=1;
//Technique which does the addition of the variables.
}
The creation price for this will likely be 147,373 fuel. If we optimize it as talked about above, that is the way it will look:
contract IntComparison {
int256 public a=-55;
uint256 public b=2;
uint256 public c=1;
//Technique which does the addition of the variables.
}
The creation price this time will likely be 131,632 fuel, 10% much less than the earlier case.
Take into account that within the first state of affairs, we had been solely making a easy contract with none advanced features. Such features would possibly require sort conversions, which might result in larger fuel consumption.
Packing occasion variables
There are circumstances the place utilizing smaller varieties for non-public variables is really helpful. These smaller varieties ought to be used when they aren’t concerned in logic that requires Solidity to carry out extra operations. Moreover, they need to be declared in a particular order to optimize storage. By packing them right into a single 32-byte storage slot, we are able to optimize storage and obtain some fuel financial savings.
If the earlier sensible contract didn’t contain advanced computations, this optimized model utilizing packing is really helpful:
contract PackingComparison {
uint8 public c=1;
int128 public a=-55;
uint256 public b=2;
}
The creation price this time will likely be 125,523 fuel, 15% much less than the earlier case.
7. Mounted-size over dynamic variables
Mounted-size variables eat much less fuel than dynamic ones in Solidity primarily due to how the Ethereum Digital Machine (EVM) handles information storage and entry. Mounted-size variables have a predictable storage format. The EVM is aware of precisely the place every fixed-size variable is saved, permitting for environment friendly entry and storage. In distinction, dynamic variables like strings, bytes, and arrays can differ in measurement, requiring extra overhead to handle their size and placement in storage. This entails extra operations to calculate offsets and handle pointers, which will increase fuel consumption.
Though that is relevant for massive arrays and sophisticated operations, in easy circumstances, we gained’t be capable to spot any distinction.
Use The Optimizer
Allow the Solidity Compiler optimization mode! It streamlines advanced expressions, decreasing each the code measurement and execution price, which lowers the fuel wanted for contract deployment and exterior calls. It additionally specializes and inlines features. Whereas inlining can improve the code measurement, it usually permits for additional simplifications and enhanced effectivity.
Earlier than you deploy your contract, activate the optimizer when compiling utilizing:
solc –optimize –bin sourceFile.sol
By default, the optimizer will optimize the contract, assuming it’s referred to as 200 instances throughout its lifetime (extra particularly, it assumes every opcode is executed round 200 instances). In order for you the preliminary contract deployment to be cheaper and the later operate executions to be costlier, set it to –optimize-runs=1. Should you anticipate many transactions and don’t care for larger deployment price and output measurement, set –optimize-runs to a excessive quantity.
There are numerous methods for decreasing fuel consumption by optimizing Solidity code. The secret’s to pick the suitable strategies for every particular case requiring optimization. Making the best decisions can usually cut back fuel prices by as much as 50%. By making use of these optimizations, builders can improve the effectivity, efficiency, and consumer expertise of their decentralized purposes (DApps), contributing to the scalability and sustainability of Ethereum Digital Machine (EVM) networks.
As we proceed to refine these practices, the way forward for Web3 growth seems more and more promising.
Cyfrin Blog: Solidity Gas Optimization Tips