The Burden of Proof(s): Code Merkleization
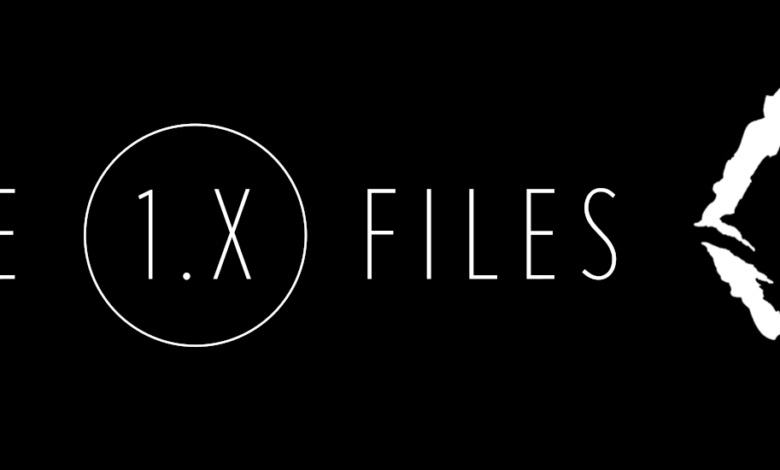
A be aware concerning the Stateless Ethereum initiative:
Analysis exercise has (understandably) slowed within the second half of 2020 as all contributors have adjusted to life on the bizarre timeline. However because the ecosystem strikes incrementally nearer to Serenity and the Eth1/Eth2 merge, Stateless Ethereum work will change into more and more related and impactful. Count on a extra substantial year-end Stateless Ethereum retrospective within the coming weeks.
Let’s roll by way of the re-cap yet another time: The final purpose of Stateless Ethereum is to take away the requirement of an Ethereum node to maintain a full copy of the up to date state trie always, and to as an alternative enable for modifications of state to depend on a (a lot smaller) piece of knowledge that proves a selected transaction is making a legitimate change. Doing this solves a significant downside for Ethereum; an issue that has up to now solely been pushed additional out by improved consumer software program: State development.
The Merkle proof wanted for Stateless Ethereum is named a ‘witness’, and it attests to a state change by offering all of the unchanged intermediate hashes required to reach at a brand new legitimate state root. Witnesses are theoretically quite a bit smaller than the complete Ethereum state (which takes 6 hours at finest to sync), however they’re nonetheless quite a bit bigger than a block (which must propagate to the entire community in just some seconds). Leaning out the dimensions of witnesses is due to this fact paramount to getting Stateless Ethereum to minimum-viable-utility.
Identical to the Ethereum state itself, quite a bit of the additional (digital) weight in witnesses comes from good contract code. If a transaction makes a name to a selected contract, the witness will by default want to incorporate the contract bytecode in its entirety with the witness. Code Merkelization is a normal method to scale back burden of good contract code in witnesses, in order that contract calls solely want to incorporate the bits of code that they ‘contact’ as a way to show their validity. With this system alone we would see a considerable discount in witness, however there are quite a bit of particulars to think about when breaking apart good contract code into byte-sized chunks.
What’s Bytecode?
There are some trade-offs to think about when splitting up contract bytecode. The query we’ll finally have to ask is “how big will the code chunks be?” – however for now, let’s take a look at some actual bytecode in a quite simple good contract, simply to know what it’s:
pragma solidity >=0.4.22 <0.7.0; contract Storage { uint256 quantity; operate retailer(uint256 num) public { quantity = num; } operate retrieve() public view returns (uint256){ return quantity; } }
When this straightforward storage contract is compiled, it turns into the machine code meant to run ‘inside’ the EVM. Right here, you’ll be able to see the identical easy storage contract proven above, however complied into particular person EVM directions (opcodes):
PUSH1 0x80 PUSH1 0x40 MSTORE CALLVALUE DUP1 ISZERO PUSH1 0xF JUMPI PUSH1 0x0 DUP1 REVERT JUMPDEST POP PUSH1 0x4 CALLDATASIZE LT PUSH1 0x32 JUMPI PUSH1 0x0 CALLDATALOAD PUSH1 0xE0 SHR DUP1 PUSH4 0x2E64CEC1 EQ PUSH1 0x37 JUMPI DUP1 PUSH4 0x6057361D EQ PUSH1 0x53 JUMPI JUMPDEST PUSH1 0x0 DUP1 REVERT JUMPDEST PUSH1 0x3D PUSH1 0x7E JUMP JUMPDEST PUSH1 0x40 MLOAD DUP1 DUP3 DUP2 MSTORE PUSH1 0x20 ADD SWAP2 POP POP PUSH1 0x40 MLOAD DUP1 SWAP2 SUB SWAP1 RETURN JUMPDEST PUSH1 0x7C PUSH1 0x4 DUP1 CALLDATASIZE SUB PUSH1 0x20 DUP2 LT ISZERO PUSH1 0x67 JUMPI PUSH1 0x0 DUP1 REVERT JUMPDEST DUP2 ADD SWAP1 DUP1 DUP1 CALLDATALOAD SWAP1 PUSH1 0x20 ADD SWAP1 SWAP3 SWAP2 SWAP1 POP POP POP PUSH1 0x87 JUMP JUMPDEST STOP JUMPDEST PUSH1 0x0 DUP1 SLOAD SWAP1 POP SWAP1 JUMP JUMPDEST DUP1 PUSH1 0x0 DUP2 SWAP1 SSTORE POP POP JUMP INVALID LOG2 PUSH5 0x6970667358 0x22 SLT KECCAK256 DUP13 PUSH7 0x1368BFFE1FF61A 0x29 0x4C CALLER 0x1F 0x5C DUP8 PUSH18 0xA3F10C9539C716CF2DF6E04FC192E3906473 PUSH16 0x6C634300060600330000000000000000
As defined in a earlier publish, these opcode directions are the essential operations of the EVM’s stack structure. They outline the easy storage contract, and all of the capabilities it accommodates. Yow will discover this contract as one of the instance solidity contracts within the Remix IDE (Word that the machine code above is an instance of the storage.sol after it is already been deployed, and never the output of the Solidity compiler, which can have some further ‘bootstrapping’ opcodes). If you happen to un-focus your eyes and picture a bodily stack machine chugging together with step-by-step computation on opcode playing cards, within the blur of the shifting stack you’ll be able to virtually see the outlines of capabilities specified by the Solidity contract.
Every time the contract receives a message name, this code runs inside each Ethereum node validating new blocks on the community. With a view to submit a legitimate transaction on Ethereum at the moment, one wants a full copy of the contract’s bytecode, as a result of working that code from starting to finish is the one option to get hold of the (deterministic) output state and related hash.
Stateless Ethereum, bear in mind, goals to vary this requirement. For instance that every one you need to do is name the operate retrieve() and nothing extra. The logic describing that operate is barely a subset of the entire contract, and on this case the EVM solely actually wants two of the basic blocks of opcode directions as a way to return the specified worth:
PUSH1 0x0 DUP1 SLOAD SWAP1 POP SWAP1 JUMP, JUMPDEST PUSH1 0x40 MLOAD DUP1 DUP3 DUP2 MSTORE PUSH1 0x20 ADD SWAP2 POP POP PUSH1 0x40 MLOAD DUP1 SWAP2 SUB SWAP1 RETURN
Within the Stateless paradigm, simply as a witness supplies the lacking hashes of un-touched state, a witness must also present the lacking hashes for un-executed items of machine code, so {that a} stateless consumer solely requires the portion of the contract it is executing.
The Code’s Witness
Good contracts in Ethereum stay in the identical place that externally-owned accounts do: as leaf nodes within the monumental single-rooted state trie. Contracts are in some ways no totally different than the externally-owned accounts people use. They’ve an deal with, can submit transactions, and maintain a stability of Ether and another token. However contract accounts are particular as a result of they have to include their very own program logic (code), or a hash thereof. One other related Merkle-Patricia Trie, known as the storageTrie retains any variables or persistent state that an energetic contract makes use of to go about its enterprise throughout execution.
This witness visualization supplies a superb sense of how necessary code merklization could possibly be in decreasing the dimensions of witnesses. See that enormous chunk of coloured squares and the way a lot greater it’s than all the opposite components within the trie? That is a single full serving of good contract bytecode.
Subsequent to it and barely under are the items of persistent state within the storageTrie, reminiscent of ERC20 stability mappings or ERC721 digital merchandise possession manifests. Since that is instance is of a witness and never a full state snapshot, these too are made principally of intermediate hashes, and solely embody the modifications a stateless consumer would require to show the subsequent block.
Code merkleization goals to separate up that enormous chunk of code, and to exchange the sector codeHash in an Ethereum account with the basis of one other Merkle Trie, aptly named the codeTrie.
Price its Weight in Hashes
Let us take a look at an instance from this Ethereum Engineering Group video, which analyzes some strategies of code chunking utilizing an ERC20 token contract. Since many of the tokens you’ve got heard of are made to the ERC-20 normal, it is a good real-world context to know code merkleization.
As a result of bytecode is lengthy and unruly, let’s use a easy shorthand of changing 4 bytes of code (8 hexidecimal characters) with both an . or X character, with the latter representing bytecode required for the execution of a selected operate (within the instance, the ERC20.switch() operate is used all through).
Within the ERC20 instance, calling the switch() operate makes use of rather less than half of the entire good contract:
XXX.XXXXXXXXXXXXXXXXXX.......................................... .....................XXXXXX..................................... ............XXXXXXXXXXXX........................................ ........................XXX.................................XX.. ......................................................XXXXXXXXXX XXXXXXXXXXXXXXXXXX...............XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX XXXXXXXXXXXXXXXXXXXXXXXXXXXXXX.................................. .......................................................XXXXXXXXX XXXXXXXXXXXXXXXXXXXXXXXXXXXXX..................................X XXXXXXXX........................................................ ....
If we wished to separate up that code into chunks of 64 bytes, solely 19 out of the 41 chunks can be required to execute a stateless switch() transaction, with the remaining of the required knowledge coming from a witness.
|XXX.XXXXXXXXXXXX|XXXXXX..........|................|................ |................|.....XXXXXX.....|................|................ |............XXXX|XXXXXXXX........|................|................ |................|........XXX.....|................|............XX.. |................|................|................|......XXXXXXXXXX |XXXXXXXXXXXXXXXX|XX..............|.XXXXXXXXXXXXXXX|XXXXXXXXXXXXXXXX |XXXXXXXXXXXXXXXX|XXXXXXXXXXXXXX..|................|................ |................|................|................|.......XXXXXXXXX |XXXXXXXXXXXXXXXX|XXXXXXXXXXXXX...|................|...............X |XXXXXXXX........|................|................|................ |....
Examine that to 31 out of 81 chunks in a 32 byte chunking scheme:
|XXX.XXXX|XXXXXXXX|XXXXXX..|........|........|........|........|........ |........|........|.....XXX|XXX.....|........|........|........|........ |........|....XXXX|XXXXXXXX|........|........|........|........|........ |........|........|........|XXX.....|........|........|........|....XX.. |........|........|........|........|........|........|......XX|XXXXXXXX |XXXXXXXX|XXXXXXXX|XX......|........|.XXXXXXX|XXXXXXXX|XXXXXXXX|XXXXXXXX |XXXXXXXX|XXXXXXXX|XXXXXXXX|XXXXXX..|........|........|........|........ |........|........|........|........|........|........|.......X|XXXXXXXX |XXXXXXXX|XXXXXXXX|XXXXXXXX|XXXXX...|........|........|........|.......X |XXXXXXXX|........|........|........|........|........|........|........ |....
On the floor it looks as if smaller chunks are extra environment friendly than bigger ones, as a result of the mostly-empty chunks are much less frequent. However right here we have to do not forget that the unused code has a value as effectively: every un-executed code chunk is changed by a hash of fastened measurement. Smaller code chunks imply a higher quantity of hashes for the unused code, and people hashes could possibly be as giant as 32 bytes every (or as small as 8 bytes). You may at this level exclaim “Hol’ up! If the hash of code chunks is a standard size of 32 bytes, how would it help to replace 32 bytes of code with 32 bytes of hash!?”.
Recall that the contract code is merkleized, that means that every one hashes are linked collectively within the codeTrie — the basis hash of which we have to validate a block. In that construction, any sequential un-executed chunks solely require one hash, irrespective of what number of there are. That’s to say, one hash can stand in for a probably giant limb full of sequential chunk hashes on the merkleized code trie, as long as none of them are required for coded execution.
We Should Gather Extra Information
The conclusion we have been constructing to is a bit of an anticlimax: There is no such thing as a theoretically ‘optimum’ scheme for code merkleization. Design decisions like fixing the dimensions of code chunks and hashes rely upon knowledge collected concerning the ‘actual world’. Each good contract will merkleize in another way, so the burden is on researchers to decide on the format that gives the most important effectivity good points to noticed mainnet exercise. What does that imply, precisely?
One factor that would point out how environment friendly a code merkleization scheme is Merkleization overhead, which solutions the query “how much extra information beyond executed code is getting included in this witness?”
Already now we have some promising results, collected utilizing a purpose-built tool developed by Horacio Mijail from Consensys’ TeamX analysis crew, which reveals overheads as small as 25% — not dangerous in any respect!
In brief, the information reveals that by-and-large smaller chunk sizes are extra environment friendly than bigger ones, particularly if smaller hashes (8-bytes) are used. However these early numbers are in no way complete, as they solely signify about 100 latest blocks. If you happen to’re studying this and involved in contributing to the Stateless Ethereum initiative by accumulating extra substantial code merkleization knowledge, come introduce your self on the ethresear.ch boards, or the #code-merkleization channel on the Eth1x/2 analysis discord!
And as all the time, when you’ve got questions, suggestions, or requests associated to “The 1.X Files” and Stateless Ethereum, DM or @gichiba on twitter.