Creating a full-stack DeFi app with Polygon
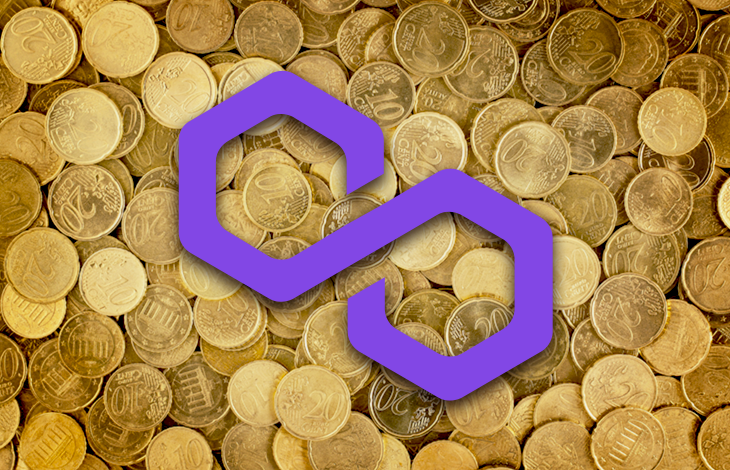
DeFi is now a main subject of dialogue within the cryptocurrency area. DeFi stands for “Decentralized finance,” which implies that there’s no central authority maintaining a tally of and controlling the switch of funds. This additionally implies that transactions in DeFi are P2P (peer to see), which implies that no central authority is answerable for transferral, and funds are despatched immediately from one entity to a different.
On this article we are going to learn to get began with DeFi by making a full-stack DeFi app on the Polygon chain utilizing Subsequent.js because the frontend. This app will promote and buy OKToken (a fictional token) from the consumer. Nevertheless, each buy transaction reduces one token from the quantity of tokens you will get per MATIC (promoting will increase this quantity by one). This isn’t a really perfect demonstration, however this manner you’ll be able to perceive easy methods to use your individual logic in Solidity sensible contracts and be taught to create your individual full-stack DeFi app utilizing Polygon.
Contents
Necessities
To get began with this tutorial, ensure you have the next:
Now that you’ve checked the necessities, let’s proceed with creating our Hardhat challenge to work with our Solidity sensible contracts.
Creating a Hardhat challenge
Navigate to a secure listing and run the next command within the terminal to initialize your Hardhat challenge:
npx hardhat
When you run the command, you must see the next Hardhat initialization wizard in your terminal.
From the record, select Create a sophisticated pattern challenge. Then you may be requested the place you need to initialize the Hardhat challenge; don’t change the sector, simply press Enter in order that the challenge will get initialized within the present listing.
Then you may be requested whether or not or not you need to set up dependencies required to your Hardhat challenge to run. Press y as a result of we will probably be needing these dependencies, and putting in them proper now’s the most effective thought.
Set up of dependencies will begin, and would possibly take a few seconds or minutes relying upon the machine you’re working. Now, run the next command within the terminal to put in one other dependency we might want to ease our Solidity contract improvement:
npm set up @openzeppelin/contracts
OpenZeppelin offers sensible contract requirements that we will use in our personal sensible contracts to simply create an Ownable, ERC-20 and ERC-721 contracts, and extra.
As soon as the dependencies are efficiently put in, open the listing in a code editor. I’ll be utilizing VS Code for this tutorial.
We will probably be creating two sensible contracts: the primary one will probably be our ERC-20 token itself and the second will probably be a vendor contract, which is able to facilitate shopping for and promoting of those tokens.
Creating our sensible contracts
Now, go to the contracts
folder and create a new Solidity file named OKToken.sol
, which is able to comprise our ERC-20 token contract.
Use the next code for this file:
// SPDX-License-Identifier: Unlicense pragma solidity ^0.8.4; import "@openzeppelin/contracts/token/ERC20/ERC20.sol"; contract OKToken is ERC20 { constructor() ERC20("OKT", "OKToken"){ _mint(msg.sender, 10000 * 10 ** 18); } }
Within the above code, we’re importing the ERC20.sol
file from @openzeppelin/contracts
which is able to assist us get began with an ERC-20 token simply. Then, within the constructor, we’re offering the image "OKT"
and title "OKToken"
for our token.
That’s all for the token contract! Now, let’s work on the seller contract. Below the contracts
folder, create a new file named OKVendor.sol
with the next code:
// SPDX-License-Identifier: MIT pragma solidity ^0.8.4; import "./OKToken.sol"; import "@openzeppelin/contracts/access/Ownable.sol"; contract OKVendor is Ownable { OKToken yourToken; uint256 public tokensPerNativeCurrency = 100; occasion BuyTokens(handle purchaser, uint256 amountOfNativeCurrency, uint256 amountOfTokens); constructor(handle tokenAddress) { yourToken = OKToken(tokenAddress); } operate buyTokens() public payable returns (uint256 tokenAmount) { require(msg.worth > 0, "You need to send some NativeCurrency to proceed"); uint256 amountToBuy = msg.worth * tokensPerNativeCurrency; uint256 vendorBalance = yourToken.balanceOf(handle(this)); require(vendorBalance >= amountToBuy, "Vendor contract has not enough tokens to perform transaction"); (bool despatched) = yourToken.switch(msg.sender, amountToBuy); require(despatched, "Failed to transfer token to user"); tokensPerNativeCurrency = tokensPerNativeCurrency - 1; emit BuyTokens(msg.sender, msg.worth, amountToBuy); return amountToBuy; } operate sellTokens(uint256 tokenAmountToSell) public { require(tokenAmountToSell > 0, "Specify an amount of token greater than zero"); uint256 userBalance = yourToken.balanceOf(msg.sender); require(userBalance >= tokenAmountToSell, "You have insufficient tokens"); uint256 amountOfNativeCurrencyToTransfer = tokenAmountToSell / tokensPerNativeCurrency; uint256 ownerNativeCurrencyBalance = handle(this).steadiness; require(ownerNativeCurrencyBalance >= amountOfNativeCurrencyToTransfer, "Vendor has insufficient funds"); (bool despatched) = yourToken.transferFrom(msg.sender, handle(this), tokenAmountToSell); require(despatched, "Failed to transfer tokens from user to vendor"); (despatched,) = msg.sender.name{worth: amountOfNativeCurrencyToTransfer}(""); tokensPerNativeCurrency = tokensPerNativeCurrency + 1; require(despatched, "Failed to send NativeCurrency to the user"); } operate getNumberOfTokensInNativeCurrency() public view returns(uint256) { return tokensPerNativeCurrency; } operate withdraw() public onlyOwner { uint256 ownerBalance = handle(this).steadiness; require(ownerBalance > 0, "No NativeCurrency present in Vendor"); (bool despatched,) = msg.sender.name{worth: handle(this).steadiness}(""); require(despatched, "Failed to withdraw"); } }
This can assist us facilitate the shopping for and promoting of tokens.
Within the above contract, first we’re importing our token contract, which we want with a view to work together with our token contract utilizing the seller contract and name capabilities.
We’re additionally importing Ownable.sol
from @openzeppelin/contracts
. Which means that the proprietor of the sensible contract can switch its possession and have entry to owners-only capabilities.
After initializing the sensible contract, we outline the variable tokensPerNativeCurrency
which states the variety of tokens which may be bought utilizing 1 MATIC. We will probably be altering this quantity based mostly on the transactions made.
We then have a constructor which is able to take OKToken’s contract handle in order that we will talk with the deployed contract and carry out capabilities on them.
Within the buyTokens()
operate, we’re performing checks to make sure the correct quantity of MATIC is shipped to the sensible contract, and that the seller contract has the required quantity of tokens. Then we name the operate switch()
from the OKToken occasion we beforehand created to switch the tokens to the request sender.
Within the sellTokens()
operate, we’re performing checks to make sure that the request sender has sufficient tokens and if the seller contract has sufficient MATIC to ship again to the request sender. Then, we use the transferFrom()
operate from the OKToken occasion we beforehand created to switch the tokens from the request sender’s pockets to the sensible contract. Nevertheless, the sender must approve this transaction; we carry out this approval on the consumer facet earlier than making the request. We are going to cowl this half once we make the entrance finish of this utility.
Lastly, we have now the withdraw()
operate, which is just accessible by the proprietor of the contracts. It permits them to withdraw all of the MATIC current on the contract.
Now that we have now the sensible contracts prepared, let’s deploy them to Polygon Mumbai testnet!
Deploying our sensible contracts
We will probably be creating a script to deploy our contract to Polygon Mumbai. As soon as the contracts are deployed, we are going to programmatically ship all of the tokens saved on the deployer’s pockets to the seller contract.
First go to hardhat.config.js
and underneath module.exports
, add the next object in order that Hardhat is aware of which community to hook up with:
networks: { mumbai: { url: " accounts: ["PRIVATE KEY HERE"], } }
We’re offering the community a title (mumbai
on this case) and offering an RPC URL. The talked about RPC URL is for Polygon Mumbai. If you wish to use Polygon Mainnet you’ll be able to choose your RPC URL. Bear in mind to enter your individual pockets non-public key with some check MATIC to pay for fuel charges concerned within the sensible contract deployment course of.
Now, underneath the scripts
folder, create a new file known as deploy.js
. Paste within the following:
const { BigNumber, utils } = require("ethers"); const hardhat = require("hardhat"); async operate fundamental() { const OKToken = await hardhat.ethers.getContractFactory("OKToken"); const oktoken = await OKToken.deploy(); await oktoken.deployed(); console.log("[📥] OKToken deployed to address: " + oktoken.handle); const OKVendor = await hardhat.ethers.getContractFactory("OKVendor"); const okvendor = await OKVendor.deploy(oktoken.handle); console.log("[📥] OKVendor deployed to address: " + okvendor.handle); await oktoken.deployed(); // Switch oktokens to vendor await oktoken.capabilities.switch(okvendor.handle, utils.parseEther("10000")); console.log("[🚀] Tokens transferred to OKVendor"); } fundamental() .then(() => course of.exit(0)) .catch((error) => { console.error(error); course of.exit(1); });
Within the above file, we’re instructing Hardhat easy methods to deploy our contract. The fundamental()
operate is the entry level right here. First, we get the OKToken
contract and deploy it. Then, we get the OKVendor
contract, present OKToken
contract handle within the constructor, and deploy the contract. Then, we switch all of the funds from OKToken
contract to OKVendor
contract.
Run the next command within the terminal to run the script and deploy our contracts to the Polygon Mumbai community:
npx hardhat run --network mumbai scripts/deploy.js --show-stack-traces
Observe that the community title should match the one talked about in hardhat.config.js
. After working the script, the contracts ought to be deployed and you must see the next in your terminal:
In the event you see an output much like this, your sensible contracts have been deployed and configured efficiently. Now, let’s proceed with creating our Subsequent.js utility.
Creating a Subsequent.js DeFi app
Below the identical listing, run the next command within the terminal to create your Subsequent.js app:
npx create-next-app frontend
The above command will create a new app and mechanically set up crucial dependencies.
Navigate to the frontend
folder and use the next command within the terminal to put in extra dependencies, which is able to assist us work together with our sensible contracts:
yarn add @thirdweb-dev/react @thirdweb-dev/sdk ethers web3
We’re putting in @thirdweb-dev/react
and @thirdweb-dev/sdk
in order that we will simply authenticate the consumer and join their wallets to our app utilizing MetaMask. ethers
is a required dependency for thirdweb so we have to set up that as properly. Lastly, we’re putting in web3
in order that we will work together with our sensible contract.
Including the thirdweb supplier
To get began, we have to wrap our app inside a thirdwebProvider
in order that thirdweb can operate correctly.
Go to your _app.js
file underneath pages
folder and add within the following:
import { thirdwebProvider, ChainId } from "@thirdweb-dev/react"; import "../styles/globals.css"; operate MyApp({ Element, pageProps }) { return ( <thirdwebProvider desiredChainId={ChainId.Mumbai}> <Element {...pageProps} /> </thirdwebProvider> ); } export default MyApp;
Within the above code, we’re importing thirdwebProvider
and enclosing our app inside it. We’re additionally offering a desiredChainId
of the chain ID of Polygon Mumbai. It’s also possible to use the chain ID for Polygon Mainnet if you want to take action.
Create a new file in your Subsequent.js app root known as contracts.js
and add the next content material:
export const oktoken = { contractAddress: "0xE83DD81890C76BB8c4b8Bc6365Ad95E5e71495E5", abi: [ { inputs: [], stateMutability: "nonpayable", kind: "constructor", }, { nameless: false, inputs: [ { indexed: true, internalType: "address", name: "owner", type: "address", }, { indexed: true, internalType: "address", name: "spender", type: "address", }, { indexed: false, internalType: "uint256", name: "value", type: "uint256", }, ], title: "Approval", kind: "event", }, { nameless: false, inputs: [ { indexed: true, internalType: "address", name: "from", type: "address", }, { indexed: true, internalType: "address", name: "to", type: "address", }, { indexed: false, internalType: "uint256", name: "value", type: "uint256", }, ], title: "Transfer", kind: "event", }, { inputs: [ { internalType: "address", name: "owner", type: "address", }, { internalType: "address", name: "spender", type: "address", }, ], title: "allowance", outputs: [ { internalType: "uint256", name: "", type: "uint256", }, ], stateMutability: "view", kind: "function", }, { inputs: [ { internalType: "address", name: "spender", type: "address", }, { internalType: "uint256", name: "amount", type: "uint256", }, ], title: "approve", outputs: [ { internalType: "bool", name: "", type: "bool", }, ], stateMutability: "nonpayable", kind: "function", }, { inputs: [ { internalType: "address", name: "account", type: "address", }, ], title: "balanceOf", outputs: [ { internalType: "uint256", name: "", type: "uint256", }, ], stateMutability: "view", kind: "function", }, { inputs: [], title: "decimals", outputs: [ { internalType: "uint8", name: "", type: "uint8", }, ], stateMutability: "view", kind: "function", }, { inputs: [ { internalType: "address", name: "spender", type: "address", }, { internalType: "uint256", name: "subtractedValue", type: "uint256", }, ], title: "decreaseAllowance", outputs: [ { internalType: "bool", name: "", type: "bool", }, ], stateMutability: "nonpayable", kind: "function", }, { inputs: [ { internalType: "address", name: "spender", type: "address", }, { internalType: "uint256", name: "addedValue", type: "uint256", }, ], title: "increaseAllowance", outputs: [ { internalType: "bool", name: "", type: "bool", }, ], stateMutability: "nonpayable", kind: "function", }, { inputs: [], title: "name", outputs: [ { internalType: "string", name: "", type: "string", }, ], stateMutability: "view", kind: "function", }, { inputs: [], title: "symbol", outputs: [ { internalType: "string", name: "", type: "string", }, ], stateMutability: "view", kind: "function", }, { inputs: [], title: "totalSupply", outputs: [ { internalType: "uint256", name: "", type: "uint256", }, ], stateMutability: "view", kind: "function", }, { inputs: [ { internalType: "address", name: "to", type: "address", }, { internalType: "uint256", name: "amount", type: "uint256", }, ], title: "transfer", outputs: [ { internalType: "bool", name: "", type: "bool", }, ], stateMutability: "nonpayable", kind: "function", }, { inputs: [ { internalType: "address", name: "from", type: "address", }, { internalType: "address", name: "to", type: "address", }, { internalType: "uint256", name: "amount", type: "uint256", }, ], title: "transferFrom", outputs: [ { internalType: "bool", name: "", type: "bool", }, ], stateMutability: "nonpayable", kind: "function", }, ], }; export const okvendor = { contractAddress: "0xAa3b8cbB24aF3EF68a0B1760704C969E57c53D7A", abi: [ { inputs: [ { internalType: "address", name: "tokenAddress", type: "address", }, ], stateMutability: "nonpayable", kind: "constructor", }, { nameless: false, inputs: [ { indexed: false, internalType: "address", name: "buyer", type: "address", }, { indexed: false, internalType: "uint256", name: "amountOfNativeCurrency", type: "uint256", }, { indexed: false, internalType: "uint256", name: "amountOfTokens", type: "uint256", }, ], title: "BuyTokens", kind: "event", }, { nameless: false, inputs: [ { indexed: true, internalType: "address", name: "previousOwner", type: "address", }, { indexed: true, internalType: "address", name: "newOwner", type: "address", }, ], title: "OwnershipTransferred", kind: "event", }, { inputs: [], title: "buyTokens", outputs: [ { internalType: "uint256", name: "tokenAmount", type: "uint256", }, ], stateMutability: "payable", kind: "function", }, { inputs: [], title: "getNumberOfTokensInNativeCurrency", outputs: [ { internalType: "uint256", name: "", type: "uint256", }, ], stateMutability: "view", kind: "function", }, { inputs: [], title: "owner", outputs: [ { internalType: "address", name: "", type: "address", }, ], stateMutability: "view", kind: "function", }, { inputs: [], title: "renounceOwnership", outputs: [], stateMutability: "nonpayable", kind: "function", }, { inputs: [ { internalType: "uint256", name: "tokenAmountToSell", type: "uint256", }, ], title: "sellTokens", outputs: [], stateMutability: "nonpayable", kind: "function", }, { inputs: [], title: "tokensPerNativeCurrency", outputs: [ { internalType: "uint256", name: "", type: "uint256", }, ], stateMutability: "view", kind: "function", }, { inputs: [ { internalType: "address", name: "newOwner", type: "address", }, ], title: "transferOwnership", outputs: [], stateMutability: "nonpayable", kind: "function", }, { inputs: [], title: "withdraw", outputs: [], stateMutability: "nonpayable", kind: "function", }, ], };
Bear in mind to switch the contract addresses with your individual in order that the Subsequent.js app tries to hook up with the right sensible contract.
Now let’s begin coding up our app. Open index.js
file underneath pages
folder and add the next:
import { useAddress, useContract, useMetamask } from "@thirdweb-dev/react"; import Head from "next/head"; import Picture from "next/image"; import { oktoken, okvendor } from "../contracts"; import kinds from "../styles/Home.module.css"; import { useEffect, useState } from "react"; import Web3 from "web3"; const web3 = new Web3(Web3.givenProvider); export default operate Residence() { const [tokensPerCurrency, setTokensPerCurrency] = useState(0); const [tokens, setTokens] = useState(0); const handle = useAddress(); const connectUsingMetamask = useMetamask(); const account = web3.defaultAccount; const buy = async () => { const contract = new web3.eth.Contract( okvendor.abi, okvendor.contractAddress ); const ethToSend = tokens / tokensPerCurrency; const buy = await contract.strategies.buyTokens().ship({ from: handle, worth: web3.utils.toWei(ethToSend.toString(), "ether"), }); console.log(buy); await fetchPrice(); }; const promote = async () => { const vendorContract = new web3.eth.Contract( okvendor.abi, okvendor.contractAddress ); const tokenContract = new web3.eth.Contract( oktoken.abi, oktoken.contractAddress ); const approve = await tokenContract.strategies .approve( okvendor.contractAddress, web3.utils.toWei(tokens.toString(), "ether") ) .ship({ from: handle, }); const sellTokens = await vendorContract.strategies.sellTokens(tokens).ship({ from: handle, }); await fetchPrice(); }; const fetchPrice = async () => { const contract = new web3.eth.Contract( okvendor.abi, okvendor.contractAddress ); const priceFromContract = await contract.strategies .getNumberOfTokensInNativeCurrency() .name(); setTokensPerCurrency(priceFromContract); }; useEffect(() => { fetchPrice(); }, []); return ( <div> <Head> <title>Change OKTokens</title> </Head> {handle ? ( <div> <p>Tokens per forex: {tokensPerCurrency}</p> <div> <enter kind="number" worth={tokens} onChange={(e) => setTokens(e.goal.worth)} /> </div> <button onClick={buy}>Buy</button> <button onClick={promote}>Promote</button> </div> ) : ( <div> <button onClick={connectUsingMetamask}>Join utilizing MetaMask</button> </div> )} </div> ); }
That is a lengthy code block, so let’s see what the code is doing step-by-step:
- Initializing the
web3
package deal utilizing a supplier arrange by thirdweb - Utilizing thirdweb hooks
useMetamask()
to authenticate anduseAddress()
to verify authentication state, then rendering the login button if the consumer doesn’t have pockets linked utilizing MetaMask - Setting varied states to map textual content containers in our app
- Creating a
fetchPrice()
operate to work together with our sensible contract and verify what number of tokens one MATIC can get, whereas additionally creating anuseEffect
to verify this worth every time the web page hundreds - Creating a
buy()
operate, which initializes our vendor contract and calls thebuyTokens()
operate from the contract, then sending some MATIC alongside with this transaction. Then, we namefetchPrice()
in order that the newest worth is proven
Lastly, we’re creating a promote()
operate, which initializes each token and vendor contract. First we work together with token contract’s approve()
operate and permit the seller contract to switch funds on our behalf. We then are calling sellTokens()
operate from the seller contract to lastly promote the tokens and obtain MATIC. We’re additionally calling fetchPrice()
to get the newest worth after transaction.
Our easy DeFi app is full! You possibly can view this app in your browser by working the next command:
yarn dev
Now when you go to you must see the next display screen, and you must be capable to make transactions.
Conclusion
This was a easy tutorial on easy methods to create your individual full-stack DeFi app based mostly on Polygon. You possibly can implement your individual logic on the sensible contracts to make it even higher relying in your group. I recommend tinkering round with the code so as to be taught in one of the best ways attainable.
Be a part of organizations like Bitso and Coinsquare who use LogRocket to proactively monitor their Web3 apps
Shopper-side points that affect customers’ potential to activate and transact in your apps can drastically have an effect on your backside line. In the event you’re excited about monitoring UX points, mechanically surfacing JavaScript errors, and monitoring sluggish community requests and part load time, try LogRocket.https://logrocket.com/signup/
LogRocket is like a DVR for net and cellular apps, recording every part that occurs in your net app or website. As a substitute of guessing why issues occur, you’ll be able to combination and report on key frontend efficiency metrics, replay consumer classes alongside with utility state, log community requests, and mechanically floor all errors.
Modernize the way you debug net and cellular apps — Start monitoring for free.